Git Maven
Everything you want to know and we might be able to share about git, GitHub, GitLab, etc.
This is a new site. We are currently moving pages over from the main Code Maven site.
Git
Two series of slides: Introduction to Git and Using Git. The first is new, the second is more detailed. Then follow by Collaborative Development and Open Source Projects.
You can also buy the book: Collaborative Development using Git and GitHub.
Collab dev series
The Git series
Git on MS Windows
Other articles
- Filtering GitHub pull requests waiting for me
- Minimal setup for Coverage report at Coveralls for Python projects hosted on GitHub
- Why Git is better than Subversion for Open Source Projects
- Merge only one commit from a Pull-request on GitHub
Getting Help for Git
Besides just typing your question in your favorite search engine, there are several places right on your command-line that can provide you help with the commands of git.
$ git help # listing the most important commands
$ git help COMMAND # man page or local web page
$ git COMMAND --help # the same
$ git help help # help about the help system
$ git help --all # list all the git commands
$ git help tutorial # a simple git tutorial
Creating a local Git repository
The power of Git comes from two main sources. Its local capabilities and its networking capabilities.
The local capabilities give you a superb version control tool. The networking capabilities allow you to collaborate with others at ease.
Let's take a look at the local capabilities.
As mentioned earlier we are going to use the command line.
Open your terminal. Navigate to a directory where you keep your projects.
For example use a directory called "projects" and inside that directory create a subdirectory for each one of your projects.
On Linux or OSX:
cd
mkdir projects
cd projects
mkdir try-git
cd try-git
On Windows it is almost the same, except that we put the "projects" directory in the root of the c:
cd /c/
mkdir projects
cd projects
mkdir try-git
cd try-git
Initialize git in the current directory
Type in the following command:
git init
The response will be something like this with the full path of the directory on your system.
Initialized empty Git repository in /Users/gabor/projects/try-git/.git/
This command created a directory called .git
(with a leading dot) that contains the "database" of Git. In general you should not change anything in that directory manually.
Using the ls
command with the appropriate flags we can see that this is the only item in our directory.
$ ls -Al
total 0
drwxr-xr-x 9 gabor staff 288 Apr 6 18:19 .git
How to get the most recent tag in a tag series?
Let's say a build process tags each commit that was used by a build and puts the build number on that tag. e.g. use tags like build-42
How do we get the most recent one?
Update the local git repository:
git checkout master
git pull
List the 5 most recent build- tags:
git tag -l 'build-*' | tail -5
The same with full sha:
git tag -l 'build-*' --format '%(refname:strip=2) %(objectname)' | tail -5
The same with short sha:
git tag -l 'build-*' --format '%(refname:strip=2) %(objectname:short)' | tail -f
git commits on one branch but not on another branch
git log one --not another
git stash - temporary saving changes
git stash saves changes temporarily. It can be useful if you are in the middle of a change and suddenly need a clean working directory.
For example, you are in the middle of fixing a bug when you notice a typo in the very same spot where you are making the changes. You could quickly change the typo as well and later commit it together with the bug-fix, but then you are mixing two unrelated changes. Not a good practice. Later, when someone reads the change and wants to understand it, this mix will be confusing. It will be unclear. Was that typo fix really part of the bug fix? A better approach would be to separate the two fixes.
You could keep working on the bug creating a mental note that you'll have to fix the typo later on, but mental notes tend to disappear with time. You could also use git stash
to push aside all the changes you made so far, make the typo fix in a clean workspace, then bring back the changes from the stash and keep working on the bug.
The way you do this:
$ git stash
will save all the changes in all the tracked file in a temporary commit in your local git repository and restore the files to the state they were in after the most recent real commit.
The response of git stash
looks like this:
$ git stash
Saved working directory and index state WIP on master: 271c4b5 MESSAGE OF THE MOST RECENT COMMIT
With the appropriate SHA1 and the message of the most recent commit at the end of response.
Then you make the typo fix. Commit it. Then you run
$ git stash pop
this will bring back the changes that were stashed away by the most recent git stash
. The changes saved in the stash will be merged back with changes made since you stashed them away.
Naturally, if there are change on the very same line both in the stashed change and in the change you made and committed (the typo), then this command will report a conflict and you'll have to manually resolve it. We'll discuss that later.
The response will look like this:
Auto-merging README
On branch master
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git checkout -- <file>..." to discard changes in working directory)
modified: README
no changes added to commit (use "git add" and/or "git commit -a")
Dropped refs/stash@{0} (7f14ba3a7afa71a7b61df8afdd059542b4761c76)
The stash is a stack
While you are fixing the typo you might notice another issue which is even more urgent than fixing the typo. Don't worry, you can run git stash
multiple times and it will save the current changes in the stash in separate entries. It handles the whole stash as a stack, meaning that if you called git stash
several time then git pop
will bring back the changes from the most recent git stash
command. (LIFO).
Listing the content of the stash
The command git stash list
will list all the saved changes:
git stash list
stash@{0}: WIP on master: 2b71505 COMMIT_MESSAGE
stash@{1}: WIP on master: 2b71505 COMMIT_MESSAGE
The most recently created stash has ID 0.
Showing the content of a stash
We can also see what is in a stash without trying to restore it. We just need to pass the ID of the stash:
$ git stash show 1
README | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
$ git stash show 0
README | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
Unfortunately in the above case both set of changes look the same from the outside. Both changed the README file in one line.
We can pass the format parameters of git diff
, for example -p
:
$ git stash show -p 0
diff --git a/README b/README
index 10c055e..bc08efb 100644
--- a/README
+++ b/README
@@ -1,5 +1,5 @@
Line one
Line two
-Line three
+Line 3
Line 4
Line five
$ git stash show -p 1
diff --git a/README b/README
index 10c055e..a5a4aef 100644
--- a/README
+++ b/README
@@ -1,4 +1,4 @@
-Line one
+Line 1
Line two
Line three
Line 4
This will show the actual diff of the change saved in the stash and now we can see that the two stashed (0 and 1) are really different.
Without any ID git stash show
will default to the most recent stash. (stash 0)
$ git stash show
README | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
Removing an entry from the stash
There are cases, more often than I should admit, that I save changes in the stash and then forget about them. Some time later I notice that there are a bunch of entries in the stash. I look at them and either don't remember why are they there or I recognize that the specific change is not relevant any more. I can remove individual stash entries without applying them to working directory by using the git stash drop
command:
For example:
git stash drop 1
will drop the one before the most recent entry in the stash. Renumber all the other entries before it.
So if we had a git stash list
like this:
stash@{0}: WIP on submit: 2ead3e3... Add button to main page
stash@{1}: On master: 9cc0589... Fix typo in e-mail
stash@{2}: On master: 9b80575... Introduce bug #42
then
$ git stash pop 1
will result in the following git stash list
:
stash@{0}: WIP on submit: 2ead3e3... Add button to main page
stash@{1}: On master: 9b80575... Introduce bug #42
Cleaning up the stash
If you have a bunch of entries in the stash and you don't want to bother with them any more, you can use the
git stash clear
command to remove all the entries at once.
Stashing new, untracked files
There are cases when we have some new and yet untracked files in our repository and we would like to put all that aside.
For that we can use the -u
also known as --include-untracked
flag:
$ git stash -u
The only issue is that git show
will not indicate that there are untracked files in the stash.
Merging conflict in stash
$ git stash pop
Auto-merging README
CONFLICT (content): Merge conflict in README
The content of the README file (the one that had the merge conflict):
<<<<<<< Updated upstream
Line first
=======
Line 1
>>>>>>> Stashed changes
Line two
Line three
Line 4
Line five
We have to manually resolve it (decide what would we really like to have in that line and remove the conflict marks). For example we will change it to:
Line 1 first
Line two
Line three
Line 4
Line five
We can then check the status
$ git status
On branch master
Unmerged paths:
(use "git reset HEAD <file>..." to unstage)
(use "git add <file>..." to mark resolution)
both modified: README
no changes added to commit (use "git add" and/or "git commit -a")
At this point we can keep editing the file or we can commit it right away.
One thing is important to remember, because the git pop
could not cleanly apply the changes, it did not remove the changes from the stash. You can verify that by using git stash list
. You will need to manually remove it by running git stash drop
as described earlier.
date: 2018-06-28T08:07:01
Git workflow for individuals
Git is excellent for collaboration among members of a team regardless of their location, but it can also make the life of the lone developer easier. Besides, looking at possible workflows for individual developers will make it easier to understand the strategies behind the various git workflows for teams.
As a lone developer I assume you have a local repository, probably cloned from some remote repository. You use the remote repository both as a backup for your local repo and maybe you also use it as the base to release your software.
Release here can mean deploying to a server or creating a zip file of the source file and uploading it to some public server. It can mean compiling and creating jar files, or sending to the Appstore or Google Play. For our purposes that does not matter. We won't delve into the release process, only that we have a notion of something released.
Let's also assume your project has made some progress, you already have released several version.
Small bug fix or small feature
Now you need to make a small bug-fix or add a small feature that will take a few minutes, maybe an hour.
You make the changes locally.
Test it with your automated tests. (You do have tons of automated tests, don't you?)
Commit to the local master branch.
As you are already done with the fix you can not tag
it and push it out to the server.
That can start an automated release and deployment process or you might do it manually.
The point is that for such small change there is not much workflow.
Interruption in work
What if while you are working on that small change you notice a typo in the code and you'd want to fix it? No problem, you can use git stash to save the changes temporarily, make your small fix, get back from the stash.
Longer work
What if the feature you are about to add is much bigger? What if you expect it to take a few days to implement adding, removing, or just changing a few thousand lines of code?
Would you just write the whole thing, create a single commit at the end of the week, and release it that way?
This can certainly work, but what if after 4 days of great work, you have a bad few minutes and make some horrible mistakes in your change? How can you get back to the good state you had after 4 great days? You can try to press "Undo" in your editor several times, but it won't be easy to find the exact location in your Undo history. Besides you might have made changes in several files. Maybe even some environment changes outside your main editor.
In any case it is a pain.
A better approach is to have frequent commits. When I work I often commit single line changes if that makes sense. Every small change that has some value gets a commit. Adding a new function. Writing a test case. etc. It will make it much easier to go back to a known good state. Also commits are very cheap in git. The biggest obstacle I see in many places is the that people are not used to work that way. In older and more "corporate-style" version control systems making a change was hard and corporates created extra difficulties by adding all kind of processes around it.
Make sure your company does not have that kind of issues. Creating a commit should be very easy.
So called "gated check-in processes" are usually a hindrance to fast paced development and don't provide the expected value.
Gated check-in processes have some kind of requirements for every commit. For example that you include the ticket number of an open ticket. Or that you write 5 lines of explanation why did you make a change. It is better to trust the developers.
OK, so I hope we can agree that if you have a feature to implement that will take several days you should make commit often. Usually every few minutes or so.
Urgent task, other feature
What if you are now notice a typo? You can use stash again as earlier, but the fixing of the typo now will be in the middle of the changes towards that new feature. There are two issues with this: Later when you look back it will be unclear why is that typo fix there. You cannot really deploy this typo fix until the end of the feature. Unless you are ready to deploy a partially implemented feature.
While you are working on this new multi-day feature you might realize there is something more urgent to do. Maybe you receive a serious bug report or have a brilliant idea to improve your product that will only take half a day.
You can stop working on the 5-day long feature, fix the bug or implement the brilliant idea, but you'll face the same problem. Now you have a partially implemented feature on your "master" branch. Either you release the code that way or you have to finish this project before you do anything else.
There is another issue however. What if you want to abandon the feature? now you have a series of commits of this feature mixed with commits that are typo fixes, bug fixes, and brilliant ideas. Reverting and removing the changes that belong to that feature will take time and quite some energy.
I am not saying this mode of work is impossible, I am just showing the potential problems you might face.
Working in feature branches
An alternative to always working on the "master" branch is to work in feature branches. When you start working on a new feature you create a branch:
$ git branch feature-name
$ git checkout feature-name
(In short form git checkout -b feature-name
.)
You work there. Make frequent commits. After you are done with the feature, you merge the branch back to "master". Add a tag and release it.
If there is an urgent bug in the production code that needs to be fixed you stop what you are doing. You might even stash the current changes. Then you switch to the "master" branch that contains the latest released version of the code. Including the bug. you fix the bug. Commit. Tag. Release.
Assuming this is something you can do in a few minutes with a single commit. If it was a bigger urgent change then after switching to the "master" branch you'd create another feature branch, e.g.
$ git branch brilliant-idea
$ git checkout brilliant-idea
Implement that in its own branch. Merge back to "master". Tag. Release.
Then you'd go back to the feature branch:
$ git checkout feature-name
and keep working till that is finished or till you are interrupted again.
This workflow allows the separation of features. If you decide to abandon one of these projects you can simply remove the branch without interfering with the rest of the work.
Follow the master: Rebase
There is one problem however with this model.
The problem is that if you work on long lived branch and you also make other changes in separate branches that end up in "master", then after a while the two branches, "master" and "feature-name" will diverge substantially making it hard to merge them together.
In order to avoid such divergence it is best to keep rebasing all the feature branches to the latest version of "master" as it progresses.
What is rebase?
Let's assume this history in which time flows from left to right. After some older changes we created the B-branch at the C (common) SHA1. From that point both "master" made progress (M1-M4) and the feature branch made progress (B1-3).
older - C - M1 - M2 - M3 - M4
\
\ B1 - B2 - B3
We need to switch to our feature branch and then rebase to master with the following commands:
$ git checkout feature-name
$ git rebase master
The result will look like this:
older - C - M1 - M2 - M3 - M4
\
\ B1 - B2 - B3
that is, we moved the changes of the feature branch as if they started from M4.
This means the branch "feature-name" now incorporates all the history of the "master" branch and thus we eliminated all the divergence.
The rebase command will be automatic if the changes made on master and on the feature-name branch were done in different areas of the code. If there are changes in overlapping areas however, we will have merge-conflicts during the rebase. We will have to resolve these merge-conflicts as the rebase progresses.
Why is continuous rebase better than merge at the end?
Why is it better to frequently rebase the feature branch, and frequently experience the pain that goes together with resolving merge conflicts? Why wouldn't it be better to leave it till we finish the feature and do the merge only then?
This is part of what we call Continuous Integration.
The assumption is that if dealing with merge-conflicts hurts, and it does, then it is better to do many small ones than one big one at the end of the project. This approach also allows us, (in case you are alone it allows you) to notice early that changes made on the master branch are in real conflict with changes made on the feature branch.
This early recognition will allow use to make corrections to our design or correct the route we took.
Rebase before every merge
To go to the extreme, some people rebase their branch when the feature is done, just before they merge it back to "master".
This means that the merging back will be unnoticeable. If we take the above example. Assuming the branch is now ready we could merge it back to "master" with the following commands:
$ git checkout master
$ git merge feature-name
The result will look like this:
older - C - M1 - M2 - M3 - M4 - B1 - B2 - B3
You won't have a separate commit for the merge. The history of the project would look like one long straight path.
The only drawback of this approach that I can see is that now it is really hard to know which commits were part of the implementation of any given feature. For this it is important to include some identification in every commit. E.g. the number of the issue (ticket) describing the feature.
Shell scripts to demo use-cases
Branch that can be merged without any problem as master has not moved at all.
#!/bin/bash
REPO='git-demo'
if [ "$1" == "" ]
then
echo $0 DIRNAME
exit 1
fi
echo "$1"
mkdir $1
cd $1
mkdir $REPO
cd $REPO
git init
echo "[user]
name = Foo
email = foo@code-maven.com
" >> .git/config
echo "First line" > README
git add .
git commit -m "first line"
echo "Second line" >> README
git add .
git commit -m "second line"
echo "Third line" >> README
git add .
git commit -m "third line"
git branch add-button
git checkout add-button
echo "One" >> BUTTON
git add .
git commit -m "button 1"
echo "Two" >> BUTTON
git add .
git commit -m "button 2"
gitk --all
git checkout master
gitk --all
git merge add-button
gitk --all
git branch -d add-button
Branch merged with a merge commit, but without any conflict as the chanes on "master" and the changes in the branch were in different files or different areas of the same file.
#!/bin/bash
REPO='git-demo'
if [ "$1" == "" ]
then
echo $0 DIRNAME
exit 1
fi
echo "$1"
mkdir $1
cd $1
mkdir $REPO
cd $REPO
git init
echo "[user]
name = Foo
email = foo@code-maven.com
" >> .git/config
echo "First line" > README
git add .
git commit -m "first line"
echo "Second line" >> README
git add .
git commit -m "second line"
echo "Third line" >> README
git add .
git commit -m "third line"
# Create branch and make some changes there
git branch add-button
git checkout add-button
echo "One" >> BUTTON
git add .
git commit -m "button 1"
echo "Two" >> BUTTON
git add .
git commit -m "button 2"
gitk --all
git checkout master
gitk --all
# Make changes on "master" as well
echo "Fourth line" > README
git add .
git commit -m "fourth line"
gitk --all
git merge add-button -m "Merge add-button"
gitk --all
git branch -d add-button
gitk --all
Branch and rebase:
#!/bin/bash
REPO='git-demo'
if [ "$1" == "" ]
then
echo $0 DIRNAME
exit 1
fi
echo "$1"
mkdir $1
cd $1
mkdir $REPO
cd $REPO
git init
echo "[user]
name = Foo
email = foo@code-maven.com
" >> .git/config
echo "First line" > README
git add .
git commit -m "first line"
echo "Second line" >> README
git add .
git commit -m "second line"
echo "Third line" >> README
git add .
git commit -m "third line"
# Create branch and make some changes there
git branch add-button
git checkout add-button
echo "One" >> BUTTON
git add .
git commit -m "button 1"
echo "Two" >> BUTTON
git add .
git commit -m "button 2"
gitk --all
git checkout master
gitk --all
# Make changes on "master" as well
echo "Fourth line" > README
git add .
git commit -m "fourth line"
gitk --all
git checkout add-button
git rebase master
git checkout master
git merge add-button -m "Merge add-button"
gitk --all
git branch -d add-button
gitk --all
Comments
You don't need to rebase (though if you are working alone, you could, and there are advantages to that), but if you want your features nicely separated each on its own feature branch (so that --first-parent
looks like a string of features), you can enforce merge even in fast-forward situation with git merge --no-ff
(and I think it is possible to configure Git to have this behavior automatic).
BTW. I have heard about git-imerge tool that allegedly has advantages of both merge and rebase, but never used it myself.
- git
- rebase 2018-06-29T08:30:01
git config
The .gitconfig
file in my home directory:
[user] name = Foo Bar email = foo@bar.com
[alias] st = status co = checkout ci = commit
# Check for conflicts before merging. See https://code-maven.com/git-check-for-conflicts-before-merge
try = merge --no-commit --no-ff
files = log --name-only
lg = log --color --graph --pretty=format:'%Cred%h%Creset -%C(yellow)%d%Creset %s %Cgreen(%cr) %C(bold blue)<%an>%Creset' --abbrev-commit
# git impact order --topo-order
topo = log --color --topo-order --pretty=format:'%Cred%h%Creset -%C(yellow)%d%Creset %s %Cgreen(%cr) %C(bold blue)<%an>%Creset' --abbrev-commit
changed = diff-tree -r --no-commit-id --name-only
last = changed HEAD
# pull and update submodules
pullall = !git pull && git submodule update --init --recursive
plog = log --all --decorate --abbrev-commit --pretty=medium --graph
meld = difftool --dir-diff
[push] default = simple
[core] excludesfile = /Users/gabor/.gitignore
[difftool "sourcetree"] cmd = opendiff "$LOCAL" "$REMOTE" path =
[mergetool "sourcetree"] cmd = /Users/gabor/Applications/Sourcetree.app/Contents/Resources/opendiff-w.sh "$LOCAL" "$REMOTE" -ancestor "$BASE" -merge "$MERGED" trustExitCode = true
Location of the commit message template file
[commit] template = /Users/gabor/.stCommitMsg
git pull defaults to git pull --rebase
[pull] rebase = true
[diff] tool = meld
[difftool] prompt = false
[difftool "meld"] cmd = meld "$LOCAL" "$REMOTE"
Install meld for better comparing of files
sudo apt-get install meld
List of recently changed files:
git log --name-only --pretty=format:'' -n 20 | grep ^[a-zA-Z0-9] | sort | uniq
An alternative:
git log --before "2019-12-31" --after "2019-12-01" --name-only | grep -v ^commit | grep -v ^Author | grep -v ^Date: | grep -v '^ ' | grep -v
'^$' | sort | uniq
git log --since="7 days ago"
will show the log for the last 7 days.
Maybe create a shell function:
function files() {
git log --pretty=format:'' --name-only "$@" | grep \\S | sort | uniq
}
and add it to ~/.bashrc
It allows us to write expressions like
files since="7 days ago"
that will show which files have changed in the last 7 days.
git log --before "2019-12-31" --after "2019-12-01"
timestamp: 2018-07-15T20:30:01
Start Git with a local repository
Find more via the Git slides.
2020-04-28T19:30:01
GitHub
Create an account on GitHub
If you don't have one yet, create an account on GitHub.
On the Front Page of GitHub that you can see in the picture below click the big green button that says "Sign up for GitHub".
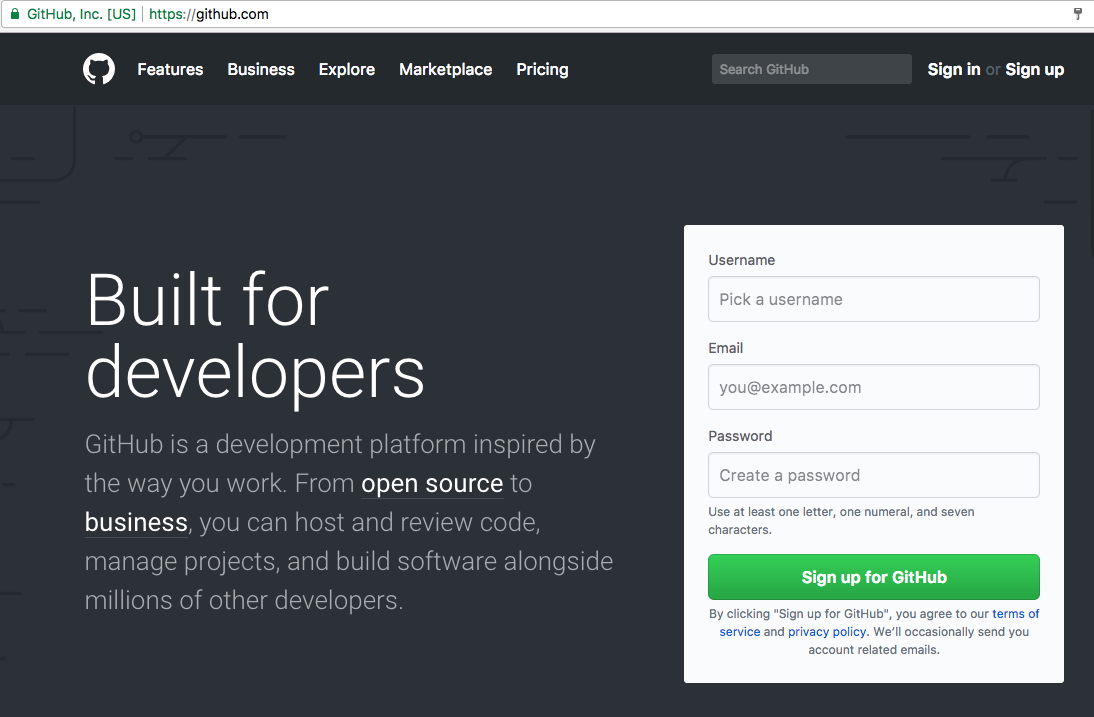
Once you click the green button you'll arrive at the "Join GitHub" page where you need to decide on a username, provide your e-mail address and pick a password. For username select something you'd like to represent you and take into account any Privacy concerns you might have. Similar with the e-mail address.
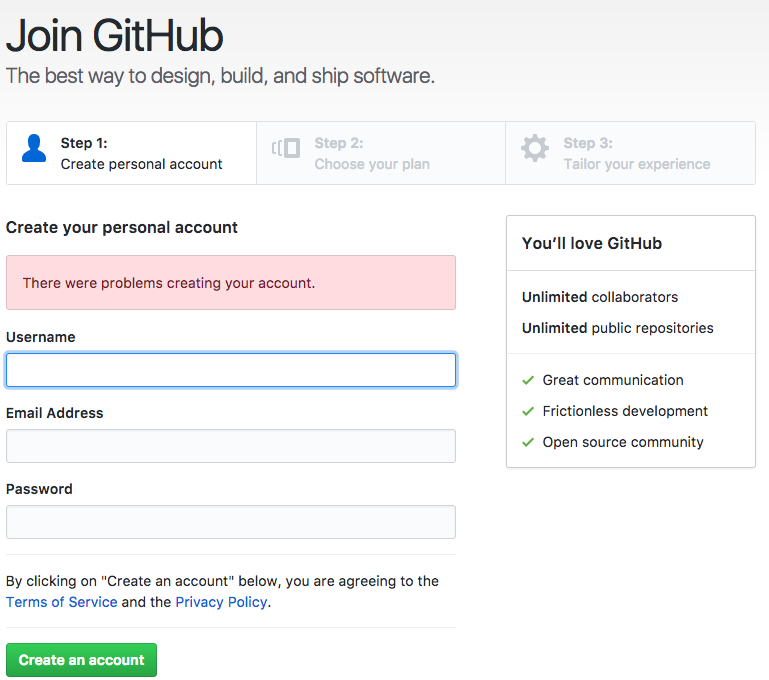
I picked szabgab-demo as the username I am going to use to demonstrate the following steps.
After clicking on the "Create an Account" button, the "Welcome to GitHub" page appears. Then select "Unlimited public repositories for free" and click on "Continue".
Actually my regular account where my username is "szabgab" is a paid account as I have a number of private repositories. For example the source code of this book is in one of these private repositories.

In the meantime I've also received an e-mail from GitHub asking to verify that the e-mail address I provided is really mine.
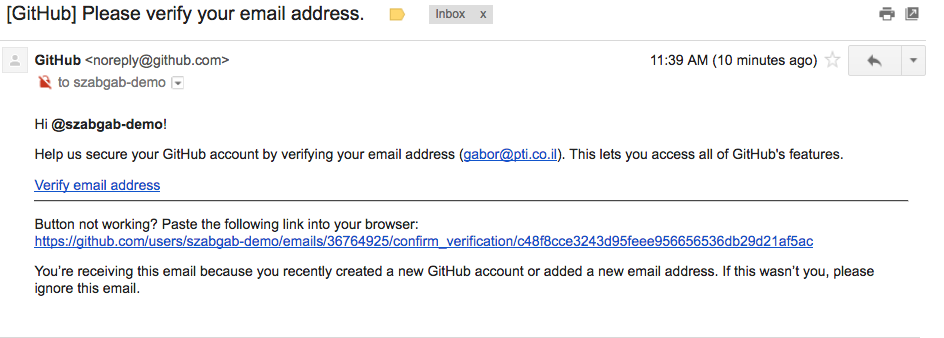
So I clicked on the long link in the email and got to a page titled "Learn Git and GitHub without any code!".
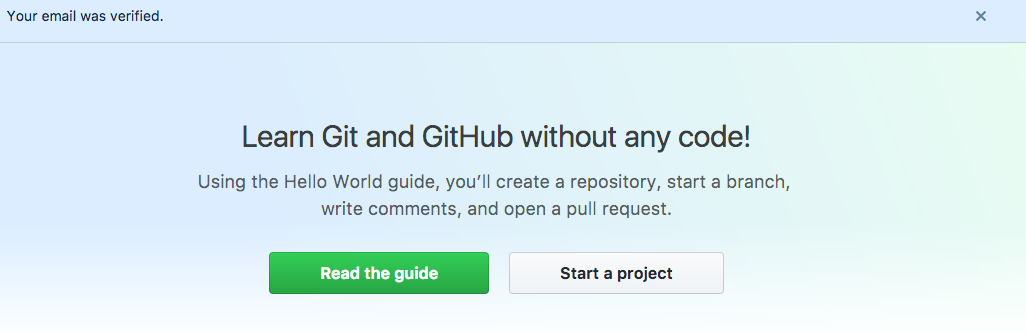
At this point you can explore what is in GitHub and how to use it on your own. When you have had enough you can come back and continue the book.
2020-03-09T07:30:01
Filtering GitHub pull requests waiting for me
When visiting GitHub it has a link to list Pull requests.
By default it uses the is:open is:pr author:szabgab
search terms which means it will show all the
pull request I (user szabgab) have opened, that are still open. This is when the (default) Created
button is pressed.
There are two other buttons: Assigned will changed the search terms to be is:open is:pr assignee:szabgab
listing
the open pull requests that were assigned to my user.
The third button is Mentioned which uses the search term is:open is:pr mentions:szabgab
which means
the pull requests where my username was mentioned as @szabgab
.
I guess I am missing some knowledge about GiHub, because what I'd really like to know is all the pull request that are waiting for my action. In other words, every pull request that were sent to any of my repositories.
It can be listed, but I could not find a button for it.
Pull requests for my repositories
In this search I used the following terms: is:open is:pr user:szabgab
. This lists all the open pull requests to any of the repositories
owned by user szabgab.
Pull requests for all of my organizations
Because I also have a few "GitHub organizations", I also wanted to be able to list all the pull requests opened to any of these organizations:
Pull request for all of my organizations
That's easy too. I just need to list all the organizations I am interested in is:open is:pr user:szabgab user:code-maven user:padreide user:perlmaven user:dwimperl
.
timestamp: 2015-10-02T10:30:01
Merge only one commit from a Pull-request on GitHub
Recently I got a pull-request for one of my projects. The pull request had two changes in it. One of them I wanted to accpet, but for the other one I had a much simpler solution and thus I wanted to only merge the change that was really good.
I am sure there is a better way than this, especially if there are more commits in that pull request and I want to cherry-pick some of them, but for now I did not need to learn that. Here is what I did:
git remote add somename https://github.com/username/repo.git
git fetch somename
git checkout somename/barnchname
git log
git show sha
git checkout master
git merge sha
git remote remove somename
git push
In details
git remote add somename https://github.com/username/repo.git
Attach the repository of the person sending the pull-request to 'somename' were I actually use the 'username' of the other person to make it easier to identify.
git fetch somename
Bring over all the changes from the repository of the other person.
git checkout somename/barnchname
Here 'somename' is the name I used to refer to the other persons repository. 'branchname' is the name of the branch the other person used for the changes in the pull request. This can be 'master' though if the other person did it well, then this will probably be some other branch name.
git log
Just looking around making sure we see the list of changes by the other person.
git show sha
Here 'sha' is the sha of the specific change I wanted to merge. (the 40-char string)
git checkout master
Switch back to my main branch.
git merge sha
Merge the specific sha. (Here too 'sha' is that 40-char string identifying the change)
git remote remove somename
Remove the connection to the remote repository of the other person.
git push
Push out to your own remote repository.
timestamp: 2015-12-26T09:30:01
Creating a website on GitHub Pages using Markdown
The address where my website was created https://szabgab.github.io/ at the end of the presentation the repository was renamed to github-pages-demo-2025-03-02 and so you can see the generated page here.
Earlier Python course with sample web sites: wis-python-course-2024-04. Specifically with that if Mazal Faraj.
The Git Maven site where I host all my git-related content.
Static site generator Jekyll is used by GitHub pages.
The github.szabgab.com web site and its source
The GitHub pages slides that I forgot to use...
I also created a repository called szabgab/experimental-repo
, but after the recording I removed it as it is not really necessary.
Transcript
1 00:00:02.250 --> 00:00:14.340 Gabor Szabo: Hello, and welcome to the Code Maven, Youtube Channel, and to your meeting especially for people, for the to the people who who are who arrive to the meeting and are going to listen to me. Live.
2 00:00:14.340 --> 00:00:33.010 Gabor Szabo: if you do that, feel free to ask questions, either by your voice or in the chat room as you like. If you are watching the video on Youtube, then, please, if you like the video, then please like it and follow the Channel. So you'll get notified on new videos.
3 00:00:33.380 --> 00:00:41.010 Gabor Szabo: My name is Gabor Sabo. I teach programming python and rust and companies.
4 00:00:41.320 --> 00:00:45.829 Gabor Szabo: and I also teach python at the Weissman Institute of Science.
5 00:00:45.940 --> 00:00:51.589 Gabor Szabo: and in that course, and this present, and it's relevant to this presentation. In this course.
6 00:00:52.670 --> 00:01:15.470 Gabor Szabo: I'm teaching Python. But because I want people to use to be able to use git, and especially Github. First, st I have this presentation about a few hours of presentation about Github and creating a website, starting with creating a website and learning markdown. Because that's part of the idea.
7 00:01:15.520 --> 00:01:31.219 Gabor Szabo: And so this video is basically this presentation is basically the 1st part of the 1st session of that course. No, no, python, no programming here, only creating a website on Github.
8 00:01:31.370 --> 00:01:34.310 Gabor Szabo: basically using a markdown.
9 00:01:34.930 --> 00:01:41.890 Gabor Szabo: I'm going to share the screen. Now, if I find the but button, I always search for it, even though it's a big and green.
10 00:01:43.180 --> 00:01:46.500 Gabor Szabo: And what you can see now hopefully, is a
11 00:01:47.510 --> 00:01:54.530 Gabor Szabo: the location of my website that doesn't exist. So you can see a 404 page.
12 00:01:54.810 --> 00:02:01.989 Gabor Szabo: And this is the address. And unfortunately, you can't probably see it, because the fonts are too small, and I
13 00:02:02.020 --> 00:02:26.289 Gabor Szabo: couldn't yet figure out how to use a magnifying glass to show this. So instead of that, I'm just going to copy, paste it here. I'm also going to put it in the chat room, actually, for those who would like to follow it later on. So this is the address of my website that I'm going to build now, as you can see, this subgo at the beginning is basically my username. And then it says, Githubio.
14 00:02:26.800 --> 00:02:43.319 Gabor Szabo: before I actually build this, and and now it's it's it. If I reload it. If you visit this page you won't see anything there, and I'm going to give you a few examples. Here is one of the repositories and that use for the course.
15 00:02:43.450 --> 00:02:53.030 Gabor Szabo: If this one was a almost a year ago where we started, and each student then creates a website for
16 00:02:53.310 --> 00:03:07.249 Gabor Szabo: herself himself. And you can visit this page. I'm also going to put this in the in the share, and I'm going to put it here as well, just in case later on I'm going to share it. I don't need this
17 00:03:07.800 --> 00:03:34.289 Gabor Szabo: ending of it. But basically you can, you can find here examples of the people built who didn't have any experience. So just to get some ideas, especially, especially, I'm going to show you one of them because I liked her her website. Okay, so this is a very nice website. I think she worked on it. I don't know a few hours, few hours, and that's it. And
18 00:03:34.300 --> 00:03:44.609 Gabor Szabo: and then there are others that use use various other things. I am going to show you another. So this is I'm sharing her link here.
19 00:03:44.640 --> 00:03:48.880 Gabor Szabo: and both in the chat room, and I'm going to show it here.
20 00:03:49.390 --> 00:03:57.550 Gabor Szabo: So here in the again. You can see. Mazalik apparently, is her username in Github. And then Githubio, is this the address?
21 00:03:57.760 --> 00:04:03.319 Gabor Szabo: And the last example I would like to show now is this one which is
22 00:04:03.620 --> 00:04:26.040 Gabor Szabo: the website that we are going to post also this video. Besides Youtube, this is the website that I recently moved over all my git related content. And this one so you can see this is a totally different address. git.codemaven.com. So codemaven is my domain, and I just
23 00:04:26.160 --> 00:04:39.789 Gabor Szabo: mapped it to be served from Github pages. So behind, the scene is basically the same. But because I have a domain, I could map it there. So what we are going to learn now is how to create a website.
24 00:04:39.790 --> 00:04:59.490 Gabor Szabo: and then it can be quite easily mapped. If you have a domain name. Then you can pick a host name, or the full domain. You can host it there. And this one is actually using Md. Books in order to generate it, not the default one that I'm going to show you. But at the end it doesn't really matter.
25 00:05:00.360 --> 00:05:05.760 Gabor Szabo: So did I share that one? Yeah, okay.
26 00:05:05.900 --> 00:05:15.079 Gabor Szabo: so what do we need in order to to build this? build this page, we're going to go to github.com.
27 00:05:15.270 --> 00:05:39.929 Gabor Szabo: Obviously, you need, and I'm going to copy this address because it's easier for me to copy paste. But then I'm just explaining it. So you already need to have an account there, of course, and if you creating an account now, this is the 1st time, then create one that is independent of everything else. So it's now, if if you're one of my students at the Weitzman Institute, then just
28 00:05:40.280 --> 00:06:04.689 Gabor Szabo: it doesn't have to be connected neither to the Institute nor to the course. If you work at some employee, it doesn't have to be connected to that. That employee. It's going to be yours, and then you can use it for various things projects, or as you work, it doesn't really matter. It should be probably yours. So mine is subgo, and I'm using it on for all kind of of things. That's my username. Now you have your user.
29 00:06:05.130 --> 00:06:13.579 Gabor Szabo: Then you create a new repository. So you're on the in this plus, I can click new repository and the name of the Repository
30 00:06:13.950 --> 00:06:35.420 Gabor Szabo: for this specific example that I'm showing needs to be this address. So your username, Dot Githubio, which is going to become the the address of your website. If if you don't do this, okay, I'm actually yeah. If you don't do this, then you'll have a
31 00:06:35.600 --> 00:06:56.769 Gabor Szabo: you can also create a website. But it won't have this this address. So basically, that's the point. So your default homepage your default page on on Github is your username dot Githubio. And then you need to create a repository with that same name. So you can see that it's user. My name username is here. And then I repeat it.
32 00:06:57.120 --> 00:07:07.949 Gabor Szabo: the repository doesn't need to be public, but there's no real point on hiding it in making it private, because at the end the content is going to be shown to the, to the
33 00:07:08.310 --> 00:07:23.749 Gabor Szabo: everyone. So why not? And especially if you'd like to show me what you did. And you'd like to get input, then make it public. And then, especially if you are one of my students, then make sure it's public. So you can get a grade for that.
34 00:07:23.850 --> 00:07:38.729 Gabor Szabo: And I started with adding the readme file, just to have some content in this repository, and I can also get ignore. It doesn't really matter, for now I can add the license. It doesn't really matter for now, and I create the repository.
35 00:07:39.170 --> 00:07:56.010 Gabor Szabo: Now, just by creating this specific repository, you can immediately click on actions, and you can see that it started to do something. It's actually it's a yellowish, brownish icon here and here it's on the right hand side. It says, queued.
36 00:07:56.270 --> 00:08:04.970 Gabor Szabo: So because this is the name of the Repository, this specific repository, it already immediately started to build your website.
37 00:08:05.240 --> 00:08:33.149 Gabor Szabo: Github created that markdown that readme file. And using that readme file, it's going to create a website, a very simple one, very simple content. But a readme file. Now, it takes some times to build it, and sometimes it doesn't update immediately. But by now you can see it's now green. And it took 37 seconds. So I spoke more than that, apparently to build the website.
38 00:08:33.480 --> 00:09:03.289 Gabor Szabo: Now, under actions, you can see this progress. So you will know when the website was built, or if there is some error. You will know this, and luckily, right now it was generated without a problem. Sometimes when I show this content. When I explain this, then Github is exactly at that time it's broken, and and then there are some failures.
39 00:09:03.970 --> 00:09:28.690 Gabor Szabo: or there could be also things that maybe we broke in the in the in the Fs we create the page. It's very unlikely, actually, so, most likely, if there is an error here in these simple cases, it's most likely some problem with the system of Github. And then you just need to wait to be fixed.
40 00:09:28.710 --> 00:09:39.830 Gabor Szabo: So anyway, this created and deployed the website. So if I go back to this URL, this tab where I had this page and reload it by pressing control. R,
41 00:09:39.910 --> 00:09:56.190 Gabor Szabo: then you will see the content of the website. So basically, that's it. Now, we have the website. I don't need anything more. Well, you probably want to improve it a little bit, so it will look a little bit better. But that's why what we are going to
42 00:09:56.730 --> 00:10:03.239 Gabor Szabo: see now. So we have a repository somewhere in one of them. Okay, this is the Repository.
43 00:10:03.340 --> 00:10:06.560 Gabor Szabo: a git repository. And then
44 00:10:07.110 --> 00:10:21.679 Gabor Szabo: we are going to build a website now, what I didn't explain here yet, basically. But I probably should that what is git. And what is Github? And so a few words about that
45 00:10:21.940 --> 00:10:32.949 Gabor Szabo: git is one of the one of the or the de facto standard version control tool these days. And what is a version control tool? When when you create a
46 00:10:32.950 --> 00:11:01.700 Gabor Szabo: basically, everyone uses some kind of versioning. Okay, even if you don't use a version control tool. Sometimes you create a document and then you create, send it to someone, and then you save it and change the name of the file, create a copy of the file and add the the date to it, or the name of the person you send to that file, and then you keep working on the original file and then send it to someone else and then create a copy again with a new date.
47 00:11:01.700 --> 00:11:14.930 Gabor Szabo: Basically, you doing version control for your file git just provides a much better tooling for this, a standardized way to do this. And Github is a cloud-based website
48 00:11:15.140 --> 00:11:43.489 Gabor Szabo: that allows you to host. These git projects basically or git based projects. And each one of these projects is called a repository. So if you go back to the main page of this repository by clicking on the address at the top. Then you can see here that it has this readme. Md. File that was created by the system. It also says that there was one. Commit one change saved.
49 00:11:43.620 --> 00:12:03.790 Gabor Szabo: and you can see the the history of it. Now, if you'd like to make some improvement. We can edit it on the website. And in this video, in this presentation, and in this video, we won't use anything else. You don't need anything on your computer. Besides this browser that we are already using. So you can click here on this
50 00:12:04.040 --> 00:12:19.579 Gabor Szabo: pencil thing that will make you allow you to edit the file. And and this is what what you have here. This is, was the default text. There, now I can change this. I can change this and say, let's say
51 00:12:20.350 --> 00:12:21.590 Gabor Szabo: welcome.
52 00:12:22.370 --> 00:12:43.879 Gabor Szabo: And then I can put here some text, some text here, just to show you some text here, and I can even go further and use various of the Markdown Syntaxes, and I'll tell you in a second or so what is marked down. But let's say I put here a enlarge the phone. So it's gonna be easier to see.
53 00:12:44.300 --> 00:12:49.350 Gabor Szabo: And Dot, and then I can put here names of. I don't know
54 00:12:53.790 --> 00:12:54.830 Gabor Szabo: fruits.
55 00:12:55.610 --> 00:13:15.550 Gabor Szabo: Okay. So as I edit. It keeps giving me this star, but I don't need any more, and I'm going to add 3 lines. And here I'm going to see. Put 2 hash marks and say, actually, here I will put 2, and I say fruits.
56 00:13:16.390 --> 00:13:21.819 Gabor Szabo: And here I'm going to say something else. What shall I put here, animals.
57 00:13:22.280 --> 00:13:33.830 Gabor Szabo: and then here, I say, one gets to you. See, I print print press, enter it automatically, gave me the number 2 dog snake.
58 00:13:34.700 --> 00:13:38.790 Gabor Szabo: I don't see the rest of it grab. Okay.
59 00:13:39.420 --> 00:14:08.290 Gabor Szabo: so far I made some changes, but now I have to save these changes, and the saving is is on. This system is called committing a change. So here, on the right hand side, you can see this big green button commit changes. I click on it. It offers me another window where I have to, when I can explain what were my changes in one sentence and then in more details. So I don't.
60 00:14:08.350 --> 00:14:24.920 Gabor Szabo: It's a good idea when you're working on a bigger project to have these explanations in this case, I don't have much to explain. I updated the readme, Md, okay, so I click here on this green button again on this other green button. This will actually do the commit.
61 00:14:24.990 --> 00:14:25.720 Gabor Szabo: And
62 00:14:25.960 --> 00:14:35.590 Gabor Szabo: basically, this saved my changes. Now, if I click on the actions. You can see that it immediately picked up the changes and started to build the website.
63 00:14:36.650 --> 00:14:54.378 Gabor Szabo: Okay, it will take it now, says it's in progress earlier. You saw it was in in queue, and it was a smaller dot here. Now it's yellowish and moving. Whatever you can see. You can learn. You learn the icons that you can see. It just makes you
64 00:14:55.210 --> 00:15:11.730 Gabor Szabo: earlier. Okay? A couple of years ago this, you didn't see this display. So we made used to make some changes, and we weren't clear when it's going to be ready. So we we went to the website and kept reloading it, and then we got annoyed because it took too long, and.
65 00:15:11.730 --> 00:15:28.150 Gabor Szabo: as you can see, last time it took 37 seconds to build the whole thing, and maybe there was some waiting time at the beginning. Now it took 41 seconds. It all depends how busy the system is. I mean the system of
66 00:15:28.150 --> 00:15:35.019 Gabor Szabo: Github. If I go back to this web, the page where my website is, and I reload it.
67 00:15:35.130 --> 00:15:50.165 Gabor Szabo: then you will see that things changed. So it displays my name. This is somehow the default you can. We can get rid of it later, but it displays my name without me telling it much, and then. But you can now see the content
68 00:15:50.770 --> 00:15:57.080 Gabor Szabo: nicely formatted that we we wrote actually.
69 00:15:57.570 --> 00:16:00.299 Gabor Szabo: before, before we we go further.
70 00:16:00.580 --> 00:16:17.699 Gabor Szabo: let me go to just one website. Okay, so let's let's even go to google.com, okay, just a generic website, or whatever. If I click on view, pay source, then you will see this.
71 00:16:18.010 --> 00:16:23.649 Gabor Szabo: Well, what can I say it? It's totally meaningless to most of us, right?
72 00:16:24.170 --> 00:16:44.849 Gabor Szabo: So this is actually HTML, and then there is some Javascript code here. But this is what generates this website. And this is a very, this is what is behind the scene and very simple thing, very simple website. At the end. I mean, the view is very simple, and but that's behind it. Now.
73 00:16:44.850 --> 00:17:00.479 Gabor Szabo: We probably probably don't want to write that that kind of stuff in order to create your page, I can even go back. It's a better example. I can go back to this web page of Mazal and see how it looks like behind the Scene View Source.
74 00:17:00.480 --> 00:17:23.579 Gabor Szabo: So here, too, I enlarge it to see. You can see there is lots of lots of this HTML, tags and whatnot, and you probably don't want to deal with this yourself. You don't want to. You just want to write the content, upload an image, write your content, and so on. So what happens here is that we can write in what.
75 00:17:23.579 --> 00:17:35.519 Gabor Szabo: in a language called Markdown. This is what we did here. So this editing that we did that I did the stars. And then the numbering. This is basically a syntax which is called Markdown.
76 00:17:36.140 --> 00:17:46.789 Gabor Szabo: And then there is a tool that takes that format and generates the HTML. So if we go to the new website and I click view source.
77 00:17:47.220 --> 00:18:06.840 Gabor Szabo: then you can see that this, too, has all kind of HTML in there. Okay, it's not that complex as the other websites. Not yet, but it already has some HTML here. And this HTML was generated by that tool based on the text I typed in
78 00:18:07.403 --> 00:18:15.200 Gabor Szabo: so the tool which is which is used by Github to generate these pages is called Jecular
79 00:18:17.310 --> 00:18:26.659 Gabor Szabo: Jekyll not and hide, but Jekyll SSG. It's the well, it's
80 00:18:26.780 --> 00:18:46.900 Gabor Szabo: it's a static site. Generator. Okay? So the generic name of these things is static static site generator or Ssg, okay, I'll copy the name here. So it's gonna be easier for everyone to understand what I'm talking about. I'm also putting it here
81 00:18:48.620 --> 00:18:59.249 Gabor Szabo: to have notes. So this is one of the hundreds, or maybe thousands, of tools that allow you to take some simple format.
82 00:18:59.340 --> 00:19:20.169 Gabor Szabo: the Markdown format that we used and generate a website from it. And Github picked specifically this tool, which is called Jekyll, which is written in Ruby, the ruby programming language which doesn't really matter for us, but just to. So, you know. So this, they used it
83 00:19:21.190 --> 00:19:33.499 Gabor Szabo: as the default tool as to generate the websites from the Markdown. We can change that if you you can change it if you like. But this is the default tool. Okay?
84 00:19:34.190 --> 00:19:42.430 Gabor Szabo: So what we look, what we saw here so far is that we could put a titles.
85 00:19:42.600 --> 00:20:07.700 Gabor Szabo: I go back actually to the page, to the source, so we can see it. If I clicked on the on the code and I click on the edit button because I'm going to change it anyway. So hash mark. And then some text is going to be with the the title of the page. Okay, a capital letters or big letters. Okay, relatively big letters.
86 00:20:07.820 --> 00:20:17.279 Gabor Szabo: Okay? Then something which has 2 hash marks is a subtitle you can see the result is slightly smaller letters.
87 00:20:18.632 --> 00:20:30.440 Gabor Szabo: I put here some text. It will be just displayed as some text what some people mentioned, that I should emphasize
88 00:20:31.570 --> 00:20:40.429 Gabor Szabo: that if I just type in the second line it won't actually show as a separate line. And this is going to be the 3rd line.
89 00:20:40.890 --> 00:20:49.740 Gabor Szabo: Okay, we'll see that basically this, these are separate paragraphs. And this is the this is going to show up as a single line.
90 00:20:49.930 --> 00:20:52.430 Gabor Szabo: Okay? The 3 dashes
91 00:20:53.410 --> 00:21:03.059 Gabor Szabo: became a horizontal line here. Apparently it also puts this, this thin lines under the title, Okay, nice.
92 00:21:03.170 --> 00:21:08.867 Gabor Szabo: And the the stores became just
93 00:21:10.230 --> 00:21:14.130 Gabor Szabo: list items. Okay, nothing. Very fancy.
94 00:21:14.570 --> 00:21:29.869 Gabor Szabo: And the numbering became these numbers. Now you might think that this was 1, 2, 3, 4, because I typed it. 1, 2, 3, 4, and this sort of right. But the question, What happens if I now remove this to dog?
95 00:21:30.548 --> 00:21:43.729 Gabor Szabo: Now it it left me with 1, 3, 4. And so let's see what happens if I save it now. Okay, so let's let's go ahead, because I really don't know. So I go ahead and commit my changes.
96 00:21:43.930 --> 00:21:52.250 Gabor Szabo: Again. I'd say, just update readme file, actually, should 2 lines.
97 00:21:53.896 --> 00:21:56.350 Gabor Szabo: Without empty
98 00:21:56.730 --> 00:22:16.730 Gabor Szabo: line. Okay, whatever. So I'm just showing you that it might be useful to add a a message. And again, what happens if I click on the actions? You can see that it's being queued right now, and it's going to be built soon. While I'm waiting for that. I would like to go back to the
99 00:22:17.200 --> 00:22:30.889 Gabor Szabo: you can go either here at the top or here to on the code part, to go back to the home page of this repository to the main page. What I wanted to show you is that now you can
100 00:22:31.580 --> 00:22:33.730 Gabor Szabo: actually, maybe I need to know
101 00:22:36.290 --> 00:22:49.889 Gabor Szabo: I'm looking for something. But I don't see it right now. Okay, interesting. You can see it was so large that it didn't show me the number of commits here. I if I put the the mouse over it.
102 00:22:49.890 --> 00:23:06.369 Gabor Szabo: then it shows 3 commits, but if I reduce the font a little bit, then it can show it will show here also in writing that is, 3 commits. So for up till now we had. We made 3, basically commits to the repository 3 changes.
103 00:23:06.970 --> 00:23:22.240 Gabor Szabo: Well, the 1st one was the creation of the of the file, and then 2 of the changes I made. I can click here and see the history of my changes. I can even click here on on the this is the id of the change.
104 00:23:22.510 --> 00:23:33.340 Gabor Szabo: and then I can see what's changed. So you can see that I removed this line and then added all these lines. This was the previous change I made.
105 00:23:34.050 --> 00:24:03.399 Gabor Szabo: I click on here. I can see. Then I added these lines and I removed the dog. Okay, so this is how we can see the history of our changes. And it might be not that important for the creation of the website when you are working alone, but when you go to a project and you start working on a project with multiple people, then it's good. It's very useful to see all the history of all the changes.
106 00:24:03.400 --> 00:24:20.800 Gabor Szabo: Who made the changes. What were the changes then? These explanations, these lines, will become even more useful, because it will give you a quick hint of of what that change was actually or what was supposed to be. Why was that made?
107 00:24:21.430 --> 00:24:23.450 Gabor Szabo: Okay? So
108 00:24:24.370 --> 00:24:31.449 Gabor Szabo: I go back to the actions just to make sure that it's finished. And as you can see, this time it was 39 seconds. So it's
109 00:24:31.730 --> 00:24:41.780 Gabor Szabo: changes and very various numbers go back to the website and reload it. And let's see what happens to the numbers 1, 2, 3, 4.
110 00:24:42.510 --> 00:24:48.390 Gabor Szabo: So the one now it became 1, 2, 3. So, despite the fact that in the file there was one.
111 00:24:48.950 --> 00:24:51.209 Gabor Szabo: What was there? I don't remember
112 00:24:55.460 --> 00:24:58.140 Gabor Szabo: in the file. If I edit the file.
113 00:24:58.890 --> 00:25:11.635 Gabor Szabo: I think it was 1, 3, 4, because I removed the 2. But on the website it shows me 1, 2, 3, which is basically the right thing to do.
114 00:25:12.330 --> 00:25:34.079 Gabor Szabo: Despite the mistake I made. I usually do actually just replace it by once. Okay, all of them. I number once, and then I don't. It doesn't matter if I rearrange the things. It won't confuse me here that I have some numbering here, and then the generated page has some different numbering. So that's 1 thing
115 00:25:34.350 --> 00:25:52.470 Gabor Szabo: the other thing we did is that we put here 2 lines, and then the 3rd line after an empty row. And if you go back to the website, you can see that the 1st 2 lines are on one line. And this is the separate line. So this is how you can have separate lines, basically.
116 00:25:54.030 --> 00:25:56.800 Gabor Szabo: and no.
117 00:25:57.140 --> 00:26:11.649 Gabor Szabo: Markdown is A is a generic language that can be used in various places in a lot of places. So that's why it's very useful, because it used in various parts of Github as well, and all kind of other things.
118 00:26:11.980 --> 00:26:31.359 Gabor Szabo: and it has flavors. So there's a default language called Markdown, but there is also a Github flavored Markdown. Search for it, and then I'm going to click the link. So this is the specification, the definition of
119 00:26:31.470 --> 00:26:32.990 Gabor Szabo: of their
120 00:26:34.570 --> 00:27:03.260 Gabor Szabo: a Github flavored Markdown language, and you can read it. Of course you can search for various things. You can see the explanations of how to do various things. The numbering here, you can see. And then you can have sub list or whatever. Okay, lots of lots of things. It's very simpler than doing. HTML, but it's still, there is stuff to learn.
121 00:27:03.520 --> 00:27:07.350 Gabor Szabo: So where is my editor now?
122 00:27:07.960 --> 00:27:29.710 Gabor Szabo: Oh, no, I want I put I put it here, and I wanted to put the link also here to save it. So I am going to add it to the below the video, they're going to have a link to my website on on the git website. And and there we're going to have this information as well listed.
123 00:27:29.840 --> 00:27:55.680 Gabor Szabo: And so what else shall I show you? There are a couple of other things that might be interesting, by the way, feel free to those people who are present, and they have the advantage of they can ask questions. So just feel free to even write in the chat things that you would like to see how to do on Github pages. Okay? And with Markdown
124 00:27:55.890 --> 00:28:16.309 Gabor Szabo: a couple of things that we might want to do while you're thinking about it. One of them is creating a link. So, for example, you might, I have this page. Right? I said, that on Github Codemaven, that's where I have all the articles. And this one. This video is going to be here as well, so I am going to
125 00:28:16.700 --> 00:28:18.520 Gabor Szabo: put here a link to
126 00:28:18.660 --> 00:28:36.080 Gabor Szabo: get Maven. This is going to be the text of the link, and it's in between square brackets. And then in between regular parentheses, I put in the link where to where this page should go.
127 00:28:36.300 --> 00:28:37.370 Gabor Szabo: Okay.
128 00:28:41.670 --> 00:29:07.299 Gabor Szabo: okay, so there is this link. That's 1 thing. Another thing is going to be some picture that we might want to add. So I'm going to answer that question in a bit that I saw. I just would like to finish this one. So let's look for for some animal. What kind of animal do we like, you know?
129 00:29:07.460 --> 00:29:13.110 Gabor Szabo: And I don't know what kind of animal should I like now, this this time?
130 00:29:22.060 --> 00:29:23.420 Gabor Szabo: a big elephant.
131 00:29:25.890 --> 00:29:30.450 Gabor Szabo: Okay, let's find images. Okay? So I have images.
132 00:29:30.984 --> 00:29:34.709 Gabor Szabo: I can click on one of them view file
133 00:29:35.180 --> 00:29:47.050 Gabor Szabo: hopefully, this will work. Yeah. So at the top, you can see this is an image, real image. I take the image link of the link of the image. And then I put it in in the
134 00:29:47.960 --> 00:29:57.649 Gabor Szabo: in here exclamation, mark, then some name. Okay, so this is a big
135 00:29:58.240 --> 00:30:13.039 Gabor Szabo: an email. Okay? And then so it looks very much like a link with a square bracket. Some text in the parentheses the address of this page where the image is, but there is an exclamation mark at the beginning.
136 00:30:13.290 --> 00:30:26.060 Gabor Szabo: So I put commit the changes, add link and image. I explain it, and I clicked already on the green button. And so it's going to be there now. It's
137 00:30:26.940 --> 00:30:40.010 Gabor Szabo: the action is building now, while it's building. I try to answer that question. So how does Github know that we are creating Github pages? Isn't it just normal repo with a readme? Md, well.
138 00:30:40.290 --> 00:30:41.790 Gabor Szabo: yes and no.
139 00:30:42.350 --> 00:30:43.370 Gabor Szabo: Yeah.
140 00:30:43.510 --> 00:30:44.840 Gabor Szabo: And
141 00:30:45.710 --> 00:30:54.739 Gabor Szabo: in the configuration of every repository. Okay, in the settings of every repository there is a place which called pages.
142 00:30:55.040 --> 00:31:01.695 Gabor Szabo: And in these pages you can say whether it's being deployed.
143 00:31:02.700 --> 00:31:07.909 Gabor Szabo: how is it being created? So let's let me create another repository, actually,
144 00:31:09.230 --> 00:31:16.250 Gabor Szabo: to see how it works. Really works. So just, I'd create a a repository called New.
145 00:31:17.920 --> 00:31:24.930 Gabor Szabo: Where is this experimental red Bull? Okay.
146 00:31:25.330 --> 00:31:29.409 Gabor Szabo: I add the readme file. And I edit.
147 00:31:29.610 --> 00:31:32.979 Gabor Szabo: okay, so let's see what happens with this one.
148 00:31:33.760 --> 00:31:40.940 Gabor Szabo: Actually the thing. The fun thing about this presentation is that I I have been doing. I did it a couple of times already, and every time
149 00:31:41.620 --> 00:31:50.389 Gabor Szabo: every time is a little different, partially because Github changes the ui and the features and whatsoe. So I got to the settings. Go to the pages.
150 00:31:50.670 --> 00:32:05.890 Gabor Szabo: and here it says deploy from branch, and it says none. So this basically is the setting when it's turned off when it's not Github pages. And if I go back to the one that
151 00:32:05.990 --> 00:32:25.129 Gabor Szabo: I was editing in this one. You can, it says, deploy from branch, and it says from the main branch and from the root folder, and there it will find the readme file. Okay? So the difference is basically that because of the name of this repository, because it has this special name.
152 00:32:25.300 --> 00:32:46.820 Gabor Szabo: Because of this, it automatically configured it to be generated to generate the Github pages. Okay, you. We can also change this one. Okay, actually, let me. Let me show you. So I can also change this one to saying, Okay, from the main branch. This rep only has a main branch and the route, and I can save it.
153 00:32:47.950 --> 00:32:53.090 Gabor Szabo: and then it will also generate a Github page. You can see it's building.
154 00:32:53.480 --> 00:33:02.159 Gabor Szabo: and then I'm going to show you in a bit what the the result is. In the meanwhile, in the meantime, the other one already finished building. Okay.
155 00:33:02.360 --> 00:33:08.720 Gabor Szabo: hopefully, actions and go back. Control. R
156 00:33:08.940 --> 00:33:18.869 Gabor Szabo: is so you can see that there is this link to get Maven. If I click on it, then I get to get Maven. So this is how I add the link. And this is the big elephant here.
157 00:33:19.030 --> 00:33:41.056 Gabor Szabo: Okay, now, we could also upload an image so we could download this file or you can have a file on your on your in your folder, and then you can upload it to your repository, and then you can serve it from the repository. How can you do this? Well, if you click here and
158 00:33:42.550 --> 00:33:55.159 Gabor Szabo: let's go. Let's 1st create a new page. Okay? So I'm first, st I would like just to create a new page. So this is the main page. The read me empty. But we can have more pages. So let's create a new file.
159 00:33:56.190 --> 00:33:58.200 Gabor Szabo: which is going to be called
160 00:33:59.210 --> 00:34:06.260 Gabor Szabo: other.md. Because I don't have better idea how to call it okay. And I put here just
161 00:34:06.600 --> 00:34:12.219 Gabor Szabo: this is the title of the other page.
162 00:34:12.400 --> 00:34:19.079 Gabor Szabo: Okay, nothing very interesting. I click on this one. And then again, I commit it.
163 00:34:19.540 --> 00:34:25.910 Gabor Szabo: Okay, so now it's going to generate it, and they will see where it it goes.
164 00:34:26.560 --> 00:34:29.270 Gabor Szabo: In the meantime we can go back to where we were
165 00:34:29.909 --> 00:34:33.930 Gabor Szabo: with the other one. Okay, so this one is the experimental repo.
166 00:34:34.770 --> 00:34:35.500 Gabor Szabo: I
167 00:34:35.630 --> 00:34:53.420 Gabor Szabo: copy the the name, so I won't have to. So I won't make a typo there. Okay, so this is called in in my user is called experimental repo, a random and basically any arbitrary name there, any repository. And then I can go to my real website.
168 00:34:53.690 --> 00:35:01.589 Gabor Szabo: Add this name here slash experimental repo. And this is where you can see the
169 00:35:01.710 --> 00:35:06.560 Gabor Szabo: content of that site. So here this
170 00:35:08.320 --> 00:35:27.069 Gabor Szabo: has nothing in there, only the this readme file is nothing besides the name, but you can see its content under the same website, under the same address with the name of the Repository, the specific repository edit here. So I'm going to put it
171 00:35:28.680 --> 00:35:36.749 Gabor Szabo: here, and I may probably going to give some explanation. I'm also adding it here, just in case you would like to see it.
172 00:35:36.910 --> 00:35:49.669 Gabor Szabo: Okay? So if yeah, I'll going to should get there, too. But here we go back actions.
173 00:35:49.850 --> 00:35:57.379 Gabor Szabo: This was already built. So the other page is also here, so I can go and type in other.
174 00:35:58.560 --> 00:36:05.490 Gabor Szabo: And now you can see the other page. So it is and address
175 00:36:07.610 --> 00:36:22.160 Gabor Szabo: inside my repository. Okay, you can see it's very similar. Both are very similar. One is just a page inside my main repository, and the other one is just another random repository.
176 00:36:22.740 --> 00:36:36.580 Gabor Szabo: So that's it. I think. One final thing, probably I would like 2 final things I would like to show, and one of them is a, let's go back to this other website, the git dot code meevant.com
177 00:36:37.377 --> 00:36:44.539 Gabor Szabo: so you might ask, how this? How does this work that I have this other address. So this one.
178 00:36:44.830 --> 00:36:56.639 Gabor Szabo: it's host, is hosted in the repository, which is actually has the same name as the website. It's it doesn't need to be okay. I only use the same name because it's easier for me.
179 00:36:57.500 --> 00:37:09.220 Gabor Szabo: What is important is that it also configured to use to create pages. So I go to the pages configuration of it. But instead of
180 00:37:09.450 --> 00:37:14.789 Gabor Szabo: and I show the other one as well, so we can see those, and they can compare the 2.
181 00:37:15.880 --> 00:37:23.030 Gabor Szabo: So this experimental thing, and also my default, page says, deploy from branch.
182 00:37:23.150 --> 00:37:34.719 Gabor Szabo: And then it says, where to deploy from this means basically to use the default. Jackie Processor. Okay, and it has
183 00:37:35.660 --> 00:37:37.000 Gabor Szabo: all kind of things
184 00:37:37.740 --> 00:38:00.210 Gabor Szabo: on this website. It says, instead of the from the deploy branch, it has used use Github actions. So actually, you could. You saw that the other one also is using github actions behind the scene. But for historical reason, it says, deploy from branch, and there are also all kinds of other things as well. But this is what happens there. So it uses Jackyl.
185 00:38:00.370 --> 00:38:22.609 Gabor Szabo: This one uses another processor which I configure, which is the Md. Book processor markdown book with Md. Book. It's called and you can see the Source code and how it's it's generated. We don't have to. We don't go into that because it's way beyond our need.
186 00:38:22.800 --> 00:38:38.159 Gabor Szabo: What is relevant. What is interesting? What might be interesting to you is that if you have a domain name you can put, you need to map your domain, name to Github pages so the world will know to arrive to Github pages.
187 00:38:38.590 --> 00:38:42.250 Gabor Szabo: And you can. You need to tell that this specific
188 00:38:43.250 --> 00:39:00.550 Gabor Szabo: repository 2 is the one that's that should serve that should be served when someone arrives to this address. So the pages generated from the Git code Maven should be served when someone arrives to this address.
189 00:39:00.600 --> 00:39:10.690 Gabor Szabo: and that's it. And then you can also say that use https, so it will be a secure address. This is only nice to have. It's not really not
190 00:39:10.690 --> 00:39:35.210 Gabor Szabo: a requirement to my students at the Institute or anyone else, you need to buy a domain name or basically rent a domain name in order to have this. But then, instead of having the Github address the Github I/O address, you will have your own address, which means that if a year from now you decide that you would like to move it to some other
191 00:39:36.078 --> 00:39:44.440 Gabor Szabo: service, and you move from Github pages to some other place, because your needs are beyond what Github can provide you.
192 00:39:45.370 --> 00:39:57.470 Gabor Szabo: Then you can just move this address to this other place, and no one really needs to know that you moved to some other place because the address this git dot code maven
193 00:39:58.110 --> 00:40:09.400 Gabor Szabo: git.codemaven.com will remain the same if you just point to a different service this different server. So it has that that advantage?
194 00:40:11.020 --> 00:40:17.269 Gabor Szabo: That's it. Any questions, any any issue that I would that you would like me to show?
195 00:40:23.170 --> 00:40:41.809 Gabor Szabo: If not, then I finish this as usual by changing the the website. So because I will, I am sure that I'm going to need to use this. Should I do this? No, I won't do this. I'll do it.
196 00:40:44.390 --> 00:41:13.380 Gabor Szabo: Sorry. I think, that I need to continue this presentation later on. But I will need to. I I do change the I'm going to change this address. So because I'm going to use, I might be able to use. I might need to use this address again to do a similar demonstration. I really like to move this repositories to some other name, so it will remain for you that you will be able to look at the repository. But
197 00:41:14.459 --> 00:41:26.609 Gabor Szabo: it won't take up this address. So what I do now is I rename this repository. So I come to this repository. I go to the settings again.
198 00:41:26.790 --> 00:41:32.940 Gabor Szabo: I say, rename it to Github pages.
199 00:41:33.290 --> 00:41:40.070 Gabor Szabo: and I never remember how to call it so. I'm need to find how my other domains are called
200 00:41:40.290 --> 00:41:47.650 Gabor Szabo: so repositories. Other repositories are called sorry Github
201 00:41:50.270 --> 00:41:58.110 Gabor Szabo: here. Okay, so this was another demo. I did. And this year this is how I where I saved this. So I'm going to
202 00:41:58.240 --> 00:42:06.629 Gabor Szabo: rename it to this one just with the current date. So the date is March. Well for us.
203 00:42:07.130 --> 00:42:22.360 Gabor Szabo: Okay, not for the people in New Zealand for us. It's March is the second march, and I click on rename. And I just need to save this. I'll click on rename. So what now happens is that if I go back to the website.
204 00:42:22.830 --> 00:42:32.689 Gabor Szabo: well, I have to wait till it's being processed right? So it's now generating the website, but because the name of the repository
205 00:42:32.690 --> 00:42:54.430 Gabor Szabo: is not the the one that I told you to to use, not the default one. Okay, so not the one that is called Myusernamegithubio. Because of that, this website is going to disappear. Okay? Because remove the repository from behind it. So I just need to wait till the processing is finished.
206 00:42:55.260 --> 00:42:56.190 Gabor Szabo: Hey?
207 00:42:57.530 --> 00:43:03.290 Gabor Szabo: Yeah, I know from New Zealand you use advanced technology. And so for you, it's already
208 00:43:04.160 --> 00:43:05.610 Gabor Szabo: the 3rd of March.
209 00:43:06.480 --> 00:43:07.520 Gabor Szabo: Hello!
210 00:43:08.940 --> 00:43:10.380 Gabor Szabo: How is the future?
211 00:43:11.040 --> 00:43:13.740 Gabor Szabo: So it's working working here.
212 00:43:14.210 --> 00:43:27.949 Gabor Szabo: If you were okay, it's done. Okay. So if I go back to the website and reload it. Now, it's a game gun. But if I put here the the address, the new address. Okay. So this Github pages demo
213 00:43:28.450 --> 00:43:31.280 Gabor Szabo: oof second of March.
214 00:43:32.020 --> 00:43:49.629 Gabor Szabo: and I load that page. That is where you can find the page. So you can find it. It's always there, even if I don't have the main website. And so also this experimental one, the one that we use the experimental. I'm going to remove this one actually, but the
215 00:43:49.810 --> 00:43:56.099 Gabor Szabo: and the other one will stay. So also. This one is here. So I I put the address of this
216 00:43:56.620 --> 00:44:07.209 Gabor Szabo: new website here, and I also going to put it here. And I'm going to link to the repository as well. So where is the repository? Let me find the repository.
217 00:44:07.580 --> 00:44:12.140 Gabor Szabo: this one, this is the repository.
218 00:44:16.150 --> 00:44:22.639 Gabor Szabo: This is where where it's being, where I saved it. And I think that's it. So
219 00:44:24.470 --> 00:44:25.879 Gabor Szabo: more than an hour.
220 00:44:27.440 --> 00:44:40.090 Gabor Szabo: Yeah, I know 1 1 thing that I really should should have shown you is how to upload the file. Okay, I'll I'll I'll still show this. So if you would. If you have an image.
221 00:44:40.880 --> 00:44:44.679 Gabor Szabo: Okay, the problem with this elephant
222 00:44:44.810 --> 00:44:55.050 Gabor Szabo: and the way we added it is that we are loading it from a different website. So if the owner of that website decides to remove the elephant, remove this image.
223 00:44:55.270 --> 00:45:00.590 Gabor Szabo: then our website will be broken. It will have an image.
224 00:45:02.090 --> 00:45:15.209 Gabor Szabo: Oh, okay, there is another question. I'll answer that one as well. But let me finish with the elephant. So if they remove the elephant the image from their website, then my website is going to be broken because it will show that there is a
225 00:45:15.350 --> 00:45:18.749 Gabor Szabo: place of for an image, but it's it's gone
226 00:45:18.900 --> 00:45:29.450 Gabor Szabo: worse than that. If they replace the elephant with something that I really don't want to show on my website. Okay? Then
227 00:45:30.150 --> 00:45:40.859 Gabor Szabo: I won't until I actually look at the page. So it automatically will start using the the new image. Okay, I won't have control over it. So it's much better to.
228 00:45:41.260 --> 00:46:00.360 Gabor Szabo: And besides, you have some copyright issues. Okay? So that's another thing. But let's say you fix the copyright issues. Okay? Who owns the image? So? But if you have your own images, okay, whatever that you would like to show, then what you can do is, you have it on your computer and then you come here.
229 00:46:00.360 --> 00:46:12.969 Gabor Szabo: Click on plus upload files. Then it will open a file selector and then you can select the file, upload the file, and just make sure it's much better if the file name
230 00:46:13.420 --> 00:46:34.720 Gabor Szabo: has only Latin letters and numbers like this, so you won't use spaces. You won't use Hebrew or Arabic, or whatever Korean letters, because it's it's much harder to to have the link, so makes the file name simple, and then you can.
231 00:46:34.940 --> 00:46:55.049 Gabor Szabo: just the same way you can embed the image. But now it's embedded from your website. So you have full control over the image and it won't disappear suddenly. Okay, so that's how you can add that image. Now there was a question, how can I remove the username link at the top.
232 00:46:55.170 --> 00:46:56.150 Gabor Szabo: so
233 00:46:56.410 --> 00:47:06.830 Gabor Szabo: that that needs a slightly longer answer. But I should have done this as well. So I'm going to do that. Show it. Now. Actually, I need to
234 00:47:07.040 --> 00:47:09.450 Gabor Szabo: and need to
235 00:47:10.050 --> 00:47:22.309 Gabor Szabo: cheat a little bit, because I never remember how to do this. But it's not not a big issue. So I have another website, which is called, I think it's called github.com.
236 00:47:22.500 --> 00:47:34.090 Gabor Szabo: which is a very defaultish website on Github, and I use it primarily to play around with this, and it is actually
237 00:47:34.200 --> 00:47:38.410 Gabor Szabo: on. And and here, yeah.
238 00:47:38.510 --> 00:47:45.989 Gabor Szabo: it is on github.com subgob, and it's called real. This is the repository. Okay.
239 00:47:46.240 --> 00:47:51.770 Gabor Szabo: I'll I'll put the link here so you can. You can find it as well if you really want.
240 00:47:52.240 --> 00:47:55.449 Gabor Szabo: This is the repository of that website.
241 00:47:56.520 --> 00:47:59.300 Gabor Szabo: And the interesting part is this one
242 00:47:59.730 --> 00:48:04.530 Gabor Szabo: that we have this config file where I can
243 00:48:04.970 --> 00:48:11.159 Gabor Szabo: put all kind of configurations. So the fact that it it showed up slightly.
244 00:48:11.480 --> 00:48:15.529 Gabor Szabo: It it was a slightly better looking website, github.
245 00:48:16.130 --> 00:48:29.470 Gabor Szabo: subgoop.com. Okay, so it just it has these colors. And whatever is because it uses the Jekyll theme caiman. There are various themes that you can use, and then
246 00:48:30.028 --> 00:48:41.240 Gabor Szabo: you can do whatever you like. Okay, so I'm going to copy this, and it's underscore config, Yaml. So I go back to the Repository
247 00:48:41.590 --> 00:48:49.689 Gabor Szabo: if I can find it, and I create the file, create new file.
248 00:48:51.720 --> 00:49:05.250 Gabor Szabo: A put this here Github Repo Github, Page, Demo, Github, page, and underscore
249 00:49:06.060 --> 00:49:19.369 Gabor Szabo: config dot Yml, Yml or Yaml is a format. Okay? That has key value pairs. And and they are using this for configuration. I commit the changes.
250 00:49:20.580 --> 00:49:25.750 Gabor Szabo: Commit them, really. And then we wait. Okay, so
251 00:49:26.170 --> 00:49:36.419 Gabor Szabo: this allows me one way to 1 1 part of the configuration. Still using the default. Jackie generator.
252 00:49:36.620 --> 00:49:40.290 Gabor Szabo: Other things that maybe I have here. I'm not sure.
253 00:49:41.832 --> 00:50:07.369 Gabor Szabo: Yes, in the assets I can also add more style sheets, and you can read about. You can create your own template. So how the web page looks. That will that involves a lot more understanding of HTML, but you can. You can. You will learn it this time. Okay, so
254 00:50:08.120 --> 00:50:16.480 Gabor Szabo: you can. You can find all kind of other things like this. Actually, I think I even had some slides for this
255 00:50:16.890 --> 00:50:19.459 Gabor Szabo: which I don't remember where I put now.
256 00:50:21.420 --> 00:50:23.880 Gabor Szabo: so I won't be able to show.
257 00:50:26.640 --> 00:50:31.400 Gabor Szabo: Let's try to find them slides.
258 00:50:31.870 --> 00:50:40.230 Gabor Szabo: Go with me then, Github pages.
259 00:50:40.500 --> 00:50:51.019 Gabor Szabo: I think I originally, I think. Yes, I wanted to use these slides, and I forgot about them to go over all the parts.
260 00:50:53.340 --> 00:50:59.600 Gabor Szabo: so I'll put the link to this, the slides here. So in case you will still want to
261 00:51:00.860 --> 00:51:03.779 Gabor Szabo: go over them, because, oh.
262 00:51:08.690 --> 00:51:16.640 Gabor Szabo: they should be the address. Yes. So I'm putting going to put here the address. These are the slides that I forgot to use now today.
263 00:51:16.810 --> 00:51:18.690 Gabor Szabo: Okay, lovely.
264 00:51:18.940 --> 00:51:30.370 Gabor Szabo: Anyway, if I go over the slides, this is created with Md. Book, and then
265 00:51:31.550 --> 00:51:38.460 Gabor Szabo: these are more, some more markdown things. I wanted to show you that some of them. I I didn't, may explain.
266 00:51:39.330 --> 00:51:40.810 Gabor Szabo: And
267 00:51:44.740 --> 00:51:49.980 Gabor Szabo: here here explanations about the layout and a little bit more about themes.
268 00:51:50.980 --> 00:51:53.399 Gabor Szabo: the configuration file I just used.
269 00:51:54.590 --> 00:52:01.019 Gabor Szabo: You can have these titles per each page and Javascript code.
270 00:52:01.390 --> 00:52:05.659 Gabor Szabo: But what I'm looking for is, oh, this one, the last page, I think
271 00:52:05.770 --> 00:52:12.889 Gabor Szabo: some examples. So here. These are mostly my websites, not all of them that use
272 00:52:13.250 --> 00:52:26.420 Gabor Szabo: static websites. Basically, they are somehow generated. Most of them are not by Jekyll, but by various tools I built. So for that, you need the programming knowledge.
273 00:52:27.130 --> 00:52:33.119 Gabor Szabo: One of the best ones is this one, or I think the best ones is a
274 00:52:34.190 --> 00:52:46.380 Gabor Szabo: a translator for the ladino language. Okay, so you type in Ladino, and it will translate to all kind of other languages, or you can type in some other language, and then it will translate it to
275 00:52:46.650 --> 00:52:48.090 Gabor Szabo: Ladino, and
276 00:52:48.270 --> 00:53:01.870 Gabor Szabo: and so on. So these are. These are examples. You can find various examples in various programming languages. And I need to update this because things have changed since I created this this page.
277 00:53:02.050 --> 00:53:27.440 Gabor Szabo: So what we were doing here is we were trying to look at this one. Yes, okay. So you can see now that your what you asked for, how can I remove the username link on the top? Well, I removed it with that configuration. There are all kind of other configurations that you you can do. And and you'll have to
278 00:53:27.660 --> 00:53:31.379 Gabor Szabo: play around basically any other questions.
279 00:53:39.220 --> 00:54:01.559 Gabor Szabo: If not, then well, please, I'll share all this stuff if you enjoyed the video or enjoyed the presentation, then please also check out the video later on and and like it and follow my channel. So you will get other videos when when I share them. If you watch the video and enjoyed it. Then please click on the like button, and also follow the channel to
280 00:54:01.680 --> 00:54:10.439 Gabor Szabo: to get notified when you, when I have a new videos, and if you'd like to join us in the in the live presentation.
281 00:54:10.560 --> 00:54:12.340 Gabor Szabo: That would be even better.
282 00:54:12.460 --> 00:54:14.480 Gabor Szabo: So thank you for.
283 00:54:15.640 --> 00:54:24.619 Gabor Szabo: Thank you for watching, and thank you for being here especially, and thank you for your questions. And I'm finishing this video. Bye, bye.
Using Git on Windows in VS Code
-
Download git and install it. Make sure to change the default editor to be "Notepad". You will thank me later.
-
In the File Explorer go to the
View
menu and make sure the heck-boxes to show file extenstion and show hidden files are checked. -
Create a file call
.gitconfig
(and not.gitignore
as I did it first) in your home directory which isc:\Users\Foo Bar
, if your name is Foo Bar.
The file hould look like this:
[user]
name = Foo Bar
email = foo@bar.com
- Clone the repository using the
https
address to a local folder that has only latin letters and underscore in its name.
Transcript
1 00:00:02.440 --> 00:00:07.940 Gabor Szabo: Hello, and welcome to the Codema Event Channel. My name is Gabor. I'm teaching
2 00:00:08.060 --> 00:00:31.820 Gabor Szabo: python, rust, git and things like this various companies. And in this video, we are going to see how to edit a Github Repository. So repository, which is located on Github. But how to do it locally using git locally and actually using visual studio code. That's what you can see on the screen. And we are doing this whole thing on windows.
3 00:00:32.090 --> 00:00:54.800 Gabor Szabo: So if you might remember, we had a video earlier where we were building Github pages, a website on Github pages using Markdown. And it was my own sort of personal website. But at the end of the video I renamed it to be called Github Pages Demo and I'm clicking on it. What you can see here is now the video about that.
4 00:00:55.510 --> 00:01:03.659 Gabor Szabo: And this is the the location of this repository. And if I want to visit it, actually, I think if I click here.
5 00:01:03.810 --> 00:01:14.649 Gabor Szabo: I forgot to do this earlier, but if I click on this one I can tell it to use the Github pages, URL, and then it will automatically select the URL.
6 00:01:14.900 --> 00:01:40.100 Gabor Szabo: And then here I can click on this one and we'll find the page. So that was the page. And it's not on my main site. But it's a subdirectory. And so we're going to edit this. We won't write programs, at least not at the beginning. We are just going to write some more markdown files. But we would like to make it locally. So we'll learn a little bit about local development.
7 00:01:40.430 --> 00:02:09.790 Gabor Szabo: So for this, we will need basically, okay, what we can do is we can start by trying to clone this repository. So here I'm already logged in into this into Github. But I'm going to use now the Https cloning method. And the reason I'm using it because that requires only my username and my password versus the Ssh. Which needs a lot more configuration, and in the long run it's probably better the Ssh version.
8 00:02:09.860 --> 00:02:27.039 Gabor Szabo: But for now I would like to prefer to show the Https version. So I clicked. Here, I take the address of this repository. Basically the way I came here, I clicked on this code. And now I'm going to the editor, the Visual Studio Code, which is already installed on my windows machine
9 00:02:27.820 --> 00:02:35.070 Gabor Szabo: and on the left hand side there is this icon which is the source control thing.
10 00:02:35.610 --> 00:02:41.999 Gabor Szabo: And now I'm trying to clone the repository from
11 00:02:42.270 --> 00:02:47.130 Gabor Szabo: Github to my own computer. Basically downloading. There's the word is cloning.
12 00:02:47.170 --> 00:02:56.390 Gabor Szabo: Now. I haven't installed git local yet. So that's why it says, download git for windows. If I click on this one hopefully, it will open
13 00:02:56.430 --> 00:03:09.080 Gabor Szabo: the website. It asked me if I want to open the website. So it's opening it. Actually, I already had it open. But it's on the Git scm website. And I need to download a
14 00:03:09.080 --> 00:03:34.219 Gabor Szabo: probably because I'm on windows. So I'm going to download it for 64 bit on windows, because that's a 64 bit machine. I mean, these days, basically, every machine is 64 bits. Actually, I don't even need to download it any, because I already did it. I just have to find the download folder. So I have it downloaded. I downloaded last week. This is the file so I can double click on it and install it.
15 00:03:35.190 --> 00:03:55.290 Gabor Szabo: It must me. And that steps through the installation. Because if there's 1 place that I need to make some changes, so here I just click on next. Unfortunately, I don't think that I can. I know how to enlarge the the fonts here so far. I just accepted the defaults next
16 00:03:55.870 --> 00:04:16.200 Gabor Szabo: next, and here. This window is where I recommend that you make some changes because well, maybe not while using the Vs code. But later on you might need this feature. So in some cases git opens an editor for yourself, and the default editor is called Vim, which is a
17 00:04:16.640 --> 00:04:32.739 Gabor Szabo: fantastic editor, but it needs like 2 years to get used to it, so I don't recommend you get started with it now. So instead of that, and this is the default of what you have here. So instead of this, I'll check here, and I will pick the use, notepad.
18 00:04:33.080 --> 00:04:50.569 Gabor Szabo: because, notepad, if you are on windows, Notepad is there all the time, and and we probably won't encounter this issue and the next, the rest are just clicking next, next, next, nothing, nothing else. Okay. So this was the only thing that I, where I made changes
19 00:04:51.840 --> 00:05:01.589 Gabor Szabo: and go to the installation. And so it's now installing. So nobody's on your windows system. And we won't really need this
20 00:05:01.690 --> 00:05:25.320 Gabor Szabo: right, not in this video. But maybe later on you'll encounter cases. And I really, really don't want you to get into vim without knowing it, because then you will be one of those millions of people who needs to know how to get out from vim. And this is one of the most popular questions on Stack overflow. Anyway, this is installing. And
21 00:05:25.760 --> 00:05:39.039 Gabor Szabo: one thing I note, I saw here that once you install it, you need to reload, so I guess I will be able to reload this with this button. Okay? So it finished the installation. I clicked on finish
22 00:05:39.450 --> 00:05:58.820 Gabor Szabo: and shows me some information about the release of git for windows. Okay, I don't really need this fine. I will have a link to this place where you can download. Git from under the video. But, as you could see, I just clicked here on this blue
23 00:05:58.820 --> 00:06:17.600 Gabor Szabo: thingy button. Yeah. So now I guess I can reload it with that link. And so visual studio had to be reloaded in order to find the installation of git, and it found it, because now I have 2, I know it, because now I have 2 blue buttons, and I can enlarge this one.
24 00:06:17.610 --> 00:06:33.989 Gabor Szabo: And so now I could open a folder. If I already have locally a git repository, then I could open that folder, but I don't have it yet. So now I need to clone the repository. I click on the clone. And here I paste that URL that I copied from
25 00:06:34.160 --> 00:06:39.100 Gabor Szabo: Github. So just to remind you again, I go back to Github.
26 00:06:39.860 --> 00:06:42.169 Gabor Szabo: If I can find this here.
27 00:06:43.420 --> 00:07:01.559 Gabor Szabo: Yeah, this one. Okay, this repository. I clicked on the green button. I picked the https and I copied this address. Okay, so this is the way I'm going to clone the repository click on Clone. I put the repository and then say, Okay, clone from Github.
28 00:07:02.895 --> 00:07:10.370 Gabor Szabo: Okay, whatever it says, there's no credentials. I hope that it won't be a problem in this video.
29 00:07:14.670 --> 00:07:23.303 Gabor Szabo: Let me try it again, Clone. From okay. Sorry. I I guess I had to click on the
30 00:07:23.630 --> 00:07:45.260 Gabor Szabo: upper link. So I go back for a second clone repository. I clicked here and earlier, I said, clone from Github. And now I clicked on this one so, and this is apparently the right one. And now it tells me, okay, please, it opens the file explorer and basically lets me find a location where I would like it to be cloned locally on my computer.
31 00:07:45.260 --> 00:08:12.209 Gabor Szabo: So I usually like to. So I don't recommend that you put it in your home directory, especially if you have a space in your name, or if you have a letter that is not the Latin letters, because that can confuse all kind of applications here. So what I do usually do, and if, when I work on windows is that I go to my C folder there I have a folder, or if I don't, then I create one which is called work.
32 00:08:12.628 --> 00:08:21.260 Gabor Szabo: can I enlarge this one? No, so just trust me if you can't see it. Well, it's called work here and in there. There. I have my projects.
33 00:08:21.960 --> 00:08:37.640 Gabor Szabo: and I think that's it. I can just select. Now select the repository destination. Okay, so this work folder is the repository destination. There are all these things here, but what this will do is we'll create a new folder.
34 00:08:38.330 --> 00:08:51.759 Gabor Szabo: so it will create a folder using the name of this repository. Would you like to open the Cloned Repository? Okay, that's the pop-up here. And yes, I would like to open it.
35 00:08:51.760 --> 00:09:18.649 Gabor Szabo: And basically it's open. It opens now the folder in the Vs code. Now, what you need to know about Vs code, if you don't know it yet, that it likes to deal with folders, and in inside the folder there are going to be files or there are files, and then it knows how to relate between those files. So that's why, instead of opening a single file, we start by opening a folder, and that's what it asked me to whether, if I would like to open this folder.
36 00:09:18.650 --> 00:09:25.350 Gabor Szabo: and that's what it did. So now it opened the folder. I'll show you also in the file, explorer, so you can see what happened.
37 00:09:25.440 --> 00:09:29.630 Gabor Szabo: And so here I can come to the C. Folder. C.
38 00:09:29.690 --> 00:09:56.990 Gabor Szabo: Disk work, and, as you can see, there is a new folder here which is the name of the Repository, the last part of the repository without my username, and so on. Now, in order to clone a repository like this, I didn't really need to be logged into github because I use the Https method, and because this repository is public, so I could access it.
39 00:09:57.700 --> 00:10:23.090 Gabor Szabo: Anyone could do this cloning, pushing back to this repository. It's not available for everyone. It's only available for people who are authenticated and who have the rights. And we'll have to get to that point in a bit, but for now you just see that there is this folder, and then here are the files. So this is the file explorer shows me. But I don't really need that now, because I can work with visual studio code.
40 00:10:23.390 --> 00:10:30.260 Gabor Szabo: And let's see now, what do we need to edit? So if I go back to the website.
41 00:10:30.370 --> 00:10:56.349 Gabor Szabo: And you might remember or not from the previous video. This is the website. And so this is the readme file. This comes from the readme file, where there is some stuff in there animals, there is this elephant, and I don't know what else. Okay, so this is in the readme file. So I'm opening the readme file. And you can see the content here. And only only thing I'm going to put here is
42 00:10:58.170 --> 00:11:10.269 Gabor Szabo: windows. Okay? So I'll just add the new entry, a title saying, Just windows, and I'm saving it. So this is what I added on my windows machine locally.
43 00:11:10.380 --> 00:11:14.790 Gabor Szabo: Now, what you can see notice here that here you got a on the
44 00:11:15.290 --> 00:11:44.960 Gabor Szabo: on the left hand side, where we had the cloning, where we have this icon of the version control, there is suddenly a blue mark. Let me try to to go back and remove these lines. Okay, if I save now. Okay, remove those lines, and the blue mark disappeared on the left hand side, because now the file is exactly the same as it was when I cloned it. I put these lines back, and once I save it. Okay, it already notices that there is some change.
45 00:11:45.170 --> 00:11:50.209 Gabor Szabo: And so now I need to. Besides saving it locally, I need to
46 00:11:50.730 --> 00:12:16.360 Gabor Szabo: commit the changes to git. So now I could. I can open this this part, and it shows me that there is one file that has changed, and now I have to save it sort of into git, and the word we use is commit to git. Okay. And so I. It already offers me to commit it. I'll click on the commit button, and
47 00:12:16.890 --> 00:12:27.599 Gabor Szabo: it tells me there are no stage changes. So the thing is that behind the scene git actually needs 2 steps in order to
48 00:12:28.060 --> 00:12:51.239 Gabor Szabo: saved in order to commit some files. The 1st step is to mark them as being staged, and then I need to commit them, and it has all kind of advantages, but we mostly disregard it, for now, because it makes life a little bit easier if it is disregarded. So it but that's what visual studio code tells me. So it says, There, there are no
49 00:12:53.030 --> 00:13:02.958 Gabor Szabo: staged changes to commit. Okay, and do you want to stage them and then say, Okay, yes, sure. So do the staging, and also the committing
50 00:13:03.620 --> 00:13:18.620 Gabor Szabo: and then now it complains. Okay, so it tells me that I need to add the configuration. I didn't configure git yet. All right. I just downloaded and installed it, and I didn't configure it, so I need to make some configuration to git.
51 00:13:19.080 --> 00:13:35.659 Gabor Szabo: That's what this error message is about. Okay, git basically wants to know my name and my email address. So when I commit into into git, it will be able to record it. And I'm going to open the git
52 00:13:35.880 --> 00:13:41.130 Gabor Szabo: log. It wants to open the git log. It's interesting. Why, I'm not sure
53 00:13:41.330 --> 00:13:47.629 Gabor Szabo: this is not what I wanted to show you. Okay, so let's try it again.
54 00:13:48.130 --> 00:14:11.189 Gabor Szabo: Commit. Okay, learn more. Okay? Basically, it needs the username and the user email. And I need to learn how to do this from visual studio code, because I don't know. So it needs to have a file in my home directory, which is called dot git config. Now on Linux and Mac. It uses this sign, the Tilde sign.
55 00:14:11.300 --> 00:14:16.909 Gabor Szabo: Okay, okay, let's met. Let me go here, on windows?
56 00:14:17.670 --> 00:14:20.550 Gabor Szabo: It explains that it's called the git config file.
57 00:14:20.780 --> 00:14:40.169 Gabor Szabo: This is the name of the file that I need to create. This is a 1 time thing that I need to do for git to prepare. And it needs to be in. You see, users and my username. Okay? So I need to create that file. I'm going to go with my file explorer to
58 00:14:40.460 --> 00:14:41.530 Gabor Szabo: see
59 00:14:41.730 --> 00:14:50.897 Gabor Szabo: users and my home directory. And here I'm going to create a new file. Okay? So new file, new.
60 00:14:52.870 --> 00:15:02.000 Gabor Szabo: text document. Okay? And it needs to be called dot git ignore, get ignore?
61 00:15:02.670 --> 00:15:04.550 Gabor Szabo: Okay it.
62 00:15:04.870 --> 00:15:13.083 Gabor Szabo: Because I changed the extension windows. Complaints. Don't worry about it. Okay, fine. I'm changing the file and
63 00:15:13.970 --> 00:15:26.709 Gabor Szabo: it can. I can see this file and this. This is something that you might need to make some changes on windows. So in the file, explorer, if you haven't done it yet. There is this view, menu at the top.
64 00:15:26.870 --> 00:15:46.649 Gabor Szabo: and there are 2 checkboxes here. One of them is to show file name extensions, so if I remove that, then it will hide the extensions. Apparently this gitignore file is still shown, so it's fine, so you will see it. But in any case I would recommend that you click on these 2 checkboxes.
65 00:15:46.960 --> 00:16:00.900 Gabor Szabo: So I have the file, and I'm going to open it, and I can open it with any editor. I'll just click on the edit and it opened it with notepad. That's fine, and let's go back to the documentation here. That explains me what I need to do.
66 00:16:02.040 --> 00:16:07.540 Gabor Szabo: and I need to, and it doesn't show it to me.
67 00:16:16.870 --> 00:16:23.875 Gabor Szabo: so I'm going to copy, paste it, and I don't. I don't find it here, so it gives me all kind of
68 00:16:24.320 --> 00:16:41.920 Gabor Szabo: ways to run this on the command line. But I wanted to avoid that. Okay, so that's why I'm not doing this. Let's see if it if it can show if it has the how it looks like. But it doesn't have it. So I'm going to open it in my
69 00:16:42.090 --> 00:16:49.520 Gabor Szabo: be under computer and then copy. I'm going to copy it from there just a second
70 00:16:50.640 --> 00:16:53.249 Gabor Szabo: while I'm copying it from the other window.
71 00:16:53.950 --> 00:17:03.230 Gabor Szabo: and I'm going to show it to you. Then, of, obviously, okay. So these are the lines that I'm going to copy now, hopefully, I'll be able to copy here.
72 00:17:11.079 --> 00:17:14.030 Gabor Szabo: And even if I can't copy a
73 00:17:14.220 --> 00:17:22.980 Gabor Szabo: no, I couldn't copy. So I just copied manually. So it it needs to say, user in square brackets. Let's can I enlarge this one?
74 00:17:23.359 --> 00:17:33.699 Gabor Szabo: Yes, fine. So it needs to say, square bracket users, and there. Here I have some indentation, and I need to put here my name, and my name is Gabel Sabo.
75 00:17:34.290 --> 00:17:38.639 Gabor Szabo: And I need to put his my email. And this say, email.
76 00:17:38.970 --> 00:17:43.790 Gabor Szabo: right? And here I put my email, subgov
77 00:17:44.270 --> 00:18:00.548 Gabor Szabo: sub gov.com. And then I save this file. Okay, control. S, save this file. Now, I'll show you something. How where it's going to end up before before you actually do this. So if I go back to this browser and
78 00:18:01.160 --> 00:18:13.050 Gabor Szabo: go back to the repository. And I look at the commits I made earlier. Okay? So that when I created this video apparently a month ago. So in each commit it, can you can see who is the author
79 00:18:13.180 --> 00:18:19.800 Gabor Szabo: and basically, let me. I don't know if if it will show you show me.
80 00:18:21.390 --> 00:18:22.150 Gabor Szabo: Huh?
81 00:18:22.290 --> 00:18:25.010 Gabor Szabo: Okay, maybe Github hides this now.
82 00:18:25.260 --> 00:18:44.330 Gabor Szabo: anyway, in each commit. If I if I look at the commit itself. Well, now it only shows the changes, but I could probably look at the the header of it, and then it will show my name and my email address. So it will be in this git repository. And then
83 00:18:44.810 --> 00:18:50.389 Gabor Szabo: and that's how it will also know who is who made the commit, anyway.
84 00:18:50.730 --> 00:19:04.169 Gabor Szabo: So put here your name and your email address and save it. And hopefully, I made it correctly. Now I can go back to the editor and try to do the commit again. Because now I made this configuration.
85 00:19:04.560 --> 00:19:12.899 Gabor Szabo: So I clicked on config. Make sure your configure username. It still doesn't know about it. Strangely.
86 00:19:13.310 --> 00:19:19.010 Gabor Szabo: Okay, why, open git log again. Get status.
87 00:19:23.820 --> 00:19:25.750 Gabor Szabo: Okay? It doesn't
88 00:19:29.290 --> 00:19:36.629 Gabor Szabo: user email name. Okay, this is what what I want. This is what I made. So I probably
89 00:19:36.740 --> 00:19:41.589 Gabor Szabo: it did. Some made some incorrect git
90 00:19:42.310 --> 00:19:46.890 Gabor Szabo: because get so no one told me.
91 00:19:47.130 --> 00:19:53.750 Gabor Szabo: While I was editing I created the file called Git Ignore, which is a totally. It's also plays. It's just a
92 00:19:54.010 --> 00:20:01.659 Gabor Szabo: error I made. There is also a file called Git Ignore, which is used. We're not using that. We need to call it. Git
93 00:20:02.060 --> 00:20:05.550 Gabor Szabo: config this file. Okay, I'll
94 00:20:05.830 --> 00:20:16.379 Gabor Szabo: copy the name now. It will also windows also complain again and let me copy the name here, so we can see it. Okay, so this is how it's called dot git config.
95 00:20:16.650 --> 00:20:23.600 Gabor Szabo: Okay, I'll also include it in in the in the video. Okay, so let's try it again commit.
96 00:20:24.340 --> 00:20:35.649 Gabor Szabo: And now it's happy. Okay? So I had to make just the right filing. That's fine. I like to make errors, because then it makes it more human, even though some people are annoyed anyway.
97 00:20:36.180 --> 00:21:05.110 Gabor Szabo: So now it asked me to give a commit message. If you remember from the previous video that when we were committing a change on the website on Github. It also asked me to to write some commit message. It already filled in. Some things here is just empty. It's not empty, but all the things that start with a hash mark will be ignored. So here I just have to add a few words just to explain what I'm doing. So I
98 00:21:05.240 --> 00:21:08.220 Gabor Szabo: add a line on
99 00:21:08.350 --> 00:21:23.450 Gabor Szabo: windows. Okay, so that's what I'm doing. Of course, if you are trying it on Mac or Linux. Then you can. You don't have to write it on the line on windows. So I just edited this file, and now I can save the file with control S, and close the file.
100 00:21:23.510 --> 00:21:30.260 Gabor Szabo: And once I do that, then get the the git client locally makes this commit.
101 00:21:30.300 --> 00:21:55.199 Gabor Szabo: Okay, so now I made this change saved it basically to get locally on my computer. But it's still not on Github. So in order to get it to Github, I need to synchronize. So basically, there are 2 commands. Either I push out to Github or I pull down from Github. Okay, so Github is the remote repository. So now I have.
102 00:21:55.270 --> 00:22:00.860 Gabor Szabo: Now, I have 2. Basically, I have 2 copies of my repository. Right? I have the one that is on Github
103 00:22:01.390 --> 00:22:23.050 Gabor Szabo: that I used earlier in the previous video, and then I cloned it locally, which made a local copy of the whole history of all the changes you can even see here all the changes shown. And so now I have local copy, and locally, I made a change. So locally, I have an additional commit here which doesn't exist on Github. Yet
104 00:22:23.190 --> 00:22:29.079 Gabor Szabo: we call this what we have on my computer, local and what we have on Github. We call it remote.
105 00:22:29.190 --> 00:22:34.509 Gabor Szabo: That's sort of the general name because of it. Of course it's removed. So now we need to.
106 00:22:35.130 --> 00:22:52.889 Gabor Szabo: In the basic terms of git, we need to push out the changes. But what visual studio code does. It? Calls it synchronizing the change which will actually 1st try to pull down any changes made on Github, which are none this time, and then we'll try to push it out.
107 00:22:53.400 --> 00:23:08.310 Gabor Szabo: If we didn't have visual studio code I would do the same manually. But now I'm trying to just click on it, and they'll try to synchronize it. And now we'll see if my previous attempts were
108 00:23:09.010 --> 00:23:36.779 Gabor Szabo: well, okay. So it managed to do this, apparently. And it's unfortunate, actually, because I wanted to show you how you have to authenticate here. So at this point, you so the story is that I already tried this, and apparently I haven't removed the authentication, so it already recognized me. If I go to the Git Repository here and I update it now, so refresh it. Control. R.
109 00:23:36.910 --> 00:23:58.270 Gabor Szabo: You will see that it there is this. Add the line on windows. So it was pushed out to get hub to github. You can also see this yellowish or brownish circle, meaning that the actions are working. Okay, and it already finished. So it's already a green, apparently. Okay.
110 00:23:58.390 --> 00:24:07.159 Gabor Szabo: right? That's that's just finished. So the website was updated. So let's go to the website. And I, if I refresh this
111 00:24:07.490 --> 00:24:16.079 Gabor Szabo: and scroll down to the end, then you can see windows. Okay, that is the line that I added locally and then push it out to Github.
112 00:24:16.880 --> 00:24:17.980 Gabor Szabo: And
113 00:24:18.290 --> 00:24:41.129 Gabor Szabo: and that's it. Okay. So now, back to the authentication part, because this will. Probably it is synchronizing, probably won't work automatically for you. So in order to be able to push out to synchronize, basically but to push out to Github. I need to be authenticated with Github. So Github needs to know that it's me.
114 00:24:41.140 --> 00:24:48.900 Gabor Szabo: My name is my username is subdop. So that's me, and it needs to know that I have the rights to push to this repository.
115 00:24:49.080 --> 00:24:50.180 Gabor Szabo: And
116 00:24:50.630 --> 00:24:56.809 Gabor Szabo: now I have the but for now that needs to mean. Know who I am? Who is the one who is pushing from here?
117 00:24:56.830 --> 00:25:20.350 Gabor Szabo: Okay, so when you click on sync, it will open you a pop up, and they'll ask you to authenticate, and there you'll have to click on it, and it will open open the browser, and then it will let you authenticate through your browser you will need to be able. You will probably need, to type in your username and password of Github in order to authenticate, and then.
118 00:25:20.350 --> 00:25:27.899 Gabor Szabo: after a couple of clicks, you will be able to. It will. It will tell you that. Okay, fine. It remembers now your password.
119 00:25:27.990 --> 00:25:32.843 Gabor Szabo: and then it will move, work smoothly. So
120 00:25:33.930 --> 00:25:37.449 Gabor Szabo: and this is what basically what happened here. So let me get
121 00:25:37.570 --> 00:25:44.139 Gabor Szabo: started more or less. Again, from the point that I
122 00:25:44.400 --> 00:26:05.769 Gabor Szabo: close this window now, okay, I don't need this visual studio code anymore. I already have cloned it locally. And so next day, okay, so let's see if we finish the day. So now I would like to make some more changes. Actually, I would like to add the file and make some more changes. So I go to my file explorer, let's say.
123 00:26:06.390 --> 00:26:08.140 Gabor Szabo: go to this project.
124 00:26:08.740 --> 00:26:16.059 Gabor Szabo: I mean, actually, I could open. I don't even need to go to the file explorer. Okay, I can just start Vs code.
125 00:26:16.160 --> 00:26:33.949 Gabor Szabo: Okay? So I'll just click on the windows key. I start Vs code and Vs code remembers the last opened folder that you had, and even the open file. Now, in case you were working on a different project, or you don't have it open, you can always come to file
126 00:26:33.950 --> 00:26:48.300 Gabor Szabo: open folder, file your file the folder where you cloned this repository locally and open it, or if you know that you've already opened it earlier. You can go to the open recent. And here you have all kind of
127 00:26:48.300 --> 00:27:02.350 Gabor Szabo: projects that you opened recently, apparently in various courses, various projects. I already use this, and it still remembers. And I need probably I need to find a way to remove all this from history. So next time I make a video. It won't show up
128 00:27:02.480 --> 00:27:16.040 Gabor Szabo: anyway. For me, it's already open. So now I can create, let's say, a new file. So let's create a new file. I think I right click, create a new file. It's called.
129 00:27:16.430 --> 00:27:23.270 Gabor Szabo: hello.md, okay, it's going to be a markdown file. And I just say, Here.
130 00:27:23.760 --> 00:27:43.810 Gabor Szabo: Hello! And from the window, from the there's actually the other file. Okay, fine from the. Read me. I wanted to link to it. And do I have a link to other. Let's search for the other keyword. No. So let's I think this is the way to Link. We'll find it out. So this is the
131 00:27:44.080 --> 00:27:50.560 Gabor Szabo: Hello, file. And I need to put here the name of the file.
132 00:27:51.210 --> 00:27:58.870 Gabor Szabo: Okay, so this is, you remember that this is how you link to some external here at the top to some external page. Fine.
133 00:27:59.280 --> 00:28:13.229 Gabor Szabo: But here, how to how we can link to an internal page. So another page. And we created this other page. This is just an another file. Okay. So now we would like to. We have them locally, and we would like to
134 00:28:13.470 --> 00:28:40.689 Gabor Szabo: add to git. Now, you can actually see that there are 2 number 2 here, because there were 2 changes that locally that are not in git yet one changes change to a file. The other one is an additional of a file. Now, you could actually play here with only committing one of them, or only committing part of them, or all kind of interesting things, but for now we just do everything at once. So we commit the whole thing again.
135 00:28:40.870 --> 00:28:56.580 Gabor Szabo: When I clicked on, commit it told me that there is nothing staged yet, so I just click on. Yes, just go ahead. Stage it. It opens again. This editor where I put in the commit message. So I put the commit message, which is
136 00:28:57.080 --> 00:28:57.659 Gabor Szabo: good,
137 00:28:58.370 --> 00:29:04.309 Gabor Szabo: New file? Okay. And I need to save this and close it here.
138 00:29:04.530 --> 00:29:30.730 Gabor Szabo: Okay? And once I did this, it made the comic locally, and here it has the local change. I can actually click here on on things and see the local changes. See the changes okay? And and what changed when and so on. It's very interesting, very useful, locally. But now again, I would like to sync the changes. So I just click it. I don't have to handle all the authentication that
139 00:29:31.660 --> 00:29:39.890 Gabor Szabo: you probably have to do it the 1st time, and I can go to the Git Repository, and
140 00:29:41.240 --> 00:29:54.600 Gabor Szabo: here it you can see it's yellowish moving, because the actions now is the Github actions. So it's building up the page, as we learned in the earlier video.
141 00:29:55.850 --> 00:29:57.770 Gabor Szabo: And and that's it.
142 00:29:57.810 --> 00:30:01.479 Gabor Szabo: Okay? So now, if you are part of my
143 00:30:01.510 --> 00:30:14.879 Gabor Szabo: course in the basement Institute, then the assignment was to create. The 1st assignment was to create a website that you can could have done on Github.
144 00:30:14.880 --> 00:30:37.120 Gabor Szabo: and the second one was actually writing some Python code and pushing it out to a repository. So for that, you need to create a new repository. Okay, that's if you want to have a separate repository for your assignments, so you create your new repository and then clone. But when you create the new repository.
145 00:30:37.120 --> 00:31:03.480 Gabor Szabo: add to add a readme file to it, so let me show before I go there. Okay, before I go there, just let's see this one. So you can see that this finished the building of the page, so I can come to this welcome page. I need to reload it again, and, as you can see, here is the link to Hello, and let's see if this works. Indeed, when I clicked on it, it went to the Hello page. Okay, that I created. So now you could see
146 00:31:03.490 --> 00:31:17.560 Gabor Szabo: how I could add, make some changes to one file, add a new file. And this is the same. If I want to add the folder, I just create a folder, add the filing into. And then when I I just
147 00:31:17.650 --> 00:31:25.849 Gabor Szabo: commit the changes, and then I synchronize them and it uploads it. Okay, so if you need to create a new repository
148 00:31:27.230 --> 00:31:35.739 Gabor Szabo: then and and work on it. Then probably what you would like is to do create a new repository, and in the repository.
149 00:31:36.290 --> 00:31:44.779 Gabor Szabo: You give it, of course, a name. You probably want to make it public if it's in the course and add the readme file. So it will have some content.
150 00:31:44.780 --> 00:32:06.939 Gabor Szabo: Actually, this git ignore that I mistakenly made earlier here. It suggests me to make that file. It's for each repository. I can create one, and it will be useful later. We'll see it. Not now. Okay, it's not not that urgent. Okay. So once you create this repository with whatever assignments or whatever name you would like to use
151 00:32:06.990 --> 00:32:24.699 Gabor Szabo: with a readme file. Then you will be able to clone it to your computer locally, and then there you can start writing a python. File your Hello, world, or whatever put it in a folder, synchronize and sync it out, and
152 00:32:24.980 --> 00:32:26.200 Gabor Szabo: and then
153 00:32:26.910 --> 00:32:47.039 Gabor Szabo: especially to my students. But even if you're just watching the video on Youtube, and you made some changes and would like me to review it then. Okay, so if you are, if you are just from the Internet, not from the course. Then you can come to the this repository specifically and open an issue.
154 00:32:47.210 --> 00:33:04.959 Gabor Szabo: And in this issue you can say, Okay, new issue. You can say, where is this repository that you would like me to review? And if you are a student in my course, then you already know, where is this repository that you should use, which is part of the course?
155 00:33:05.642 --> 00:33:06.789 Gabor Szabo: So that's it.
156 00:33:07.350 --> 00:33:12.070 Gabor Szabo: You open the issue, and then that's how I will know. Where is your?
157 00:33:12.430 --> 00:33:42.080 Gabor Szabo: Where are your files? Where are your repositories? I think that's the basics basically of using git locally. There are a lot more things you could do. I think, as I mentioned that, for example, you can click here, and then you can see the actual changes. So in this change I added these lines, and if I go back to this one, which I apparently did a long time a month ago, then it shows that these lines were added, and this line was removed.
158 00:33:42.150 --> 00:33:56.640 Gabor Szabo: and all kind of other things. So you can use this to see locally what changes were made when, and I guess also you could see by whom. Yes, so I put the mouse and waited a second, and it shows me that
159 00:33:56.640 --> 00:34:13.439 Gabor Szabo: it's Gabor Sabo that's me. Who edited. When was it changed? What was my commit message then? And so on. Okay, so you don't need to do it on Github. If you have a local clone of your whole repository and a lot more things to to do.
160 00:34:14.296 --> 00:34:15.770 Gabor Szabo: Locally. In
161 00:34:16.219 --> 00:34:27.130 Gabor Szabo: one more thing, maybe I add one line, add a line. Okay? So I just added a line to this file, and then I can come here
162 00:34:28.219 --> 00:34:48.280 Gabor Szabo: and see, yeah, right? So I just clicked on the file in the let me show you. So inside, let me close this one. Okay, I have the file edited and I clicked open the menu for the source control and then click on the file itself. And it shows me the actual change.
163 00:34:48.620 --> 00:35:09.160 Gabor Szabo: Okay, so in just notice, this is this is just showing the modifications that I added this line. Okay, so even before you make the commit, you can actually look at. Okay, what did I change? It's especially useful if you made some changes, then you left for 2 weeks vacation. And then you came back and
164 00:35:09.370 --> 00:35:26.900 Gabor Szabo: you have some local changes. And you don't remember anymore what you did. And you would like to know? Okay, what did? What were the changes I made and forgot to commit and and push out so that could be also useful. Okay, you don't need to go through 2 weeks vacation for that. I can do this in 10 min. I can forget things. So anyway.
165 00:35:27.800 --> 00:35:37.570 Gabor Szabo: that's it, I think. The basics of of using git locally and
166 00:35:37.990 --> 00:35:49.449 Gabor Szabo: with a Github project and pushing out changes. So that's it. If you watch the video and enjoyed it. Then please like it and follow the channel
167 00:35:49.600 --> 00:35:52.209 Gabor Szabo: and see you at one of the upcoming videos.
168 00:35:52.360 --> 00:35:53.470 Gabor Szabo: Bye-bye.
Create website on GitHub pages using mdBook
mdBook is a utility to create modern online books from Markdown files.
See mdBook Maven to see what we can say about it
Git on Windows
A video series where you can learn how to use Git on MS Windows.
Git on MS Windows
- Why use a version control system
- Download and install git
- Download and install Git on MS Windows
- Getting started with Git on MS Windows
- Creating a local git repository
- Adding first file to your git repository
- Getting help for git
- Exercise git session 1
- Git file status
- Recording changes in Git
For more articles and videos check out the Git page.
timestamp: 2020-05-18T07:30:01
Why use a version control system (VCS)?
Before we get into learning how to use git, let's first talk about why to use a version control system at all?
Almost everyone uses version control even if they don't use a real version control system.
Most people will save versions of whatever they are working on. For example if they work on a file called wedding_vows.doc at one point they might save it as wedding_vows_1.doc and then when they get divorced and marry again they might update the original file and now create a copy called wedding_vows_2.doc.
Then they go on and might have wedding_vows_3.doc etc.
If they have multiple files then maybe the create a copy of all the files and put them in directories called "wedding_1" and "wedding_2".
Some other people, instead of numbering the versions they attach the current date to it. So they would have a file called wedding_vows_2013_01_07.doc or a folder called wedding_2013_01_07.
They basically create a home-made, manual version control system.
If you are writing an application then usually you'll have a lot more files and you'll have a lot more versions. Then this home-made version control can easily get out of control.
Why reinvent the wheel if there are already excellent open source version control systems? Such as git?
Advantages of having a Version Control System (VCS)
There are a number of advantages using a well-known VCS.
One of them is that if you'd like someone else to start working on your project you don't need to teach them about your own VCS. Most likely they already know how to use it.
Fearless experimentation
If you are a chemist and you combine two materials, in most cases you cannot go back and separate them again. If you are doctor and cut of a part of a patient in the hope that it will solve the problem the patient has, you cannot go back. You cannot undo it.
In programming if you overwrite a file with some changes your editor might have an undo functionality, but it is usually very limited in its history.
Using a version control system a programmer could do any experiment and safely know that s/he can go back to an earlier state, an earlier version of the code.
Fearless deletion
In many cases I see people keep around old code saying: ok maybe we'll need it at some point. Currently we are not using this code but maybe some day need to copy-paste from it or maybe literally we'll need to use it, so we don't delete it.
Then you accumulate a lot of, well, basically garbage.
Code that's not used that not only takes up space and compilation time, but take up a lot of brain cycles (mental energy) when someone actually read you code an encounters this function and tries to understand it. The person might spend a lot of time trying to figure out what does this function do till they realize that the function is not in use. Or maybe it is in use by something else which is not in use. So it can get complicated.
If you have a version control system like git or some other version control system then you can just delete this code, commit your changes to your version control system and at any point of time when you need it you can go back this version, look at it copy from it pieces, or just bring back the whole piece of code in case you now need it.
Easier (smoother) collaboration
Another thing is the easier and quite smooth collaboration with other people.
As long as you work alone you don't need collaboration, of course, but once you do collaborate, having a version control system is extremely important.
Let's go back to the example without version control system.
You have a file. You make some changes. Another person has the same file. That person also makes some changes.
How can you now combine (merge together) the two changes?
How can you make sure that the changes of both of you are kept?
In many organization there is a shared disk and people take files from there and save it back. What if two people try to edit the file at the same file? Either the system limits that only one person can open the file at one time, but that means people have to wait one for the other. Or if there is no locking then if both edit and save the file then the second one wins. All the changes made by the person who saved first will be lost.
Having a version control system can make this really smooth
Of course it is not perfect and there still might be some issue. The people still have to talk to each other, but in many cases it makes it a lot smoother to collaborate with other people. Even if they are in different time-zones, different locations
History
One of the basic thing is that you can easily look at the history of your code base. What changed were made, if there multiple people contributing to the source code then who made the changes.
If you are keeping a good track of why you made the change, so every time when you make a change you have some explanation why did you do that then it's a gold mine.
Actually I rarely need to look at the history, but when you do, it's extremely important, it is extremely valuable that you can look at the history.
It is a little-bit like backups. You really rarely need to have backups when you do, if you don't have it then it is a really big issue.
So this is more or less similar to history although we'll look a lot more at the history just because we all kinds of small mistakes.
So for example, one of the mistakes that we can easily make:
You have some working code and then you make some changes, other people make some changes and check the parts that you changes. Two weeks later someone reports that some feature stopped working.
They know it used to work. You remember it used to work, but you see that it does not work now.
You may remember that it worked two weeks ago. How can you find out what happened? Which change broke it?
Version control systems in general and git specifically have tools for it, but without having this history we really can't do anything.
Having the history you'll be able to track down which change caused this error? Why was that change made? Usually these changes are made for some good purpose, but maybe they have some side-effects. You will have to understand that and not just revert the change, because that will break something else.
Which Version Control System (VCS)?
Finally, just to get to end of the slide, there are bunch of version control systems.
Some of them are proprietary so you have to buy them, many of them are open source. There is a list of them on the slide: git, mercurial (hg), subversion (svn), cvs, rcs, ... that's just going back in history. The most popular these days is git. Most popular by far.
It is a distributed version control system, it was created by Linus Torvalds the same person who created the Linux Operating System (actually the Linux kernel), It is used by almost everyone. Both Open Source and corporate.
So that's what you are going to learn later on in this course.
timestamp: 2020-05-07T21:00:12
Download and install git
This time we are going to talk about downloading and installing git. There is going to be a separate episode on how to install on Windows, this is some generic overview.
All the slides and the specific slides about git installation.
Linux
For RedHat, CentOS and similar yum-based Linux distributions:
sudo yum install git-core
For Ubuntu, Debian, and other apt- or deb-based Linux distributions:
sudo apt-get install git-core
You could also download the latest version of git from git-scm, but usually you are better off using the version that is packaged by the vendor of your Linux distribution. It is usually better to use the standard package-management system of your Linux distribution.
Of course, if you have a really, really old version of Linux then, ..., then yous should probably upgrade your Linux.
Well even in older versions of Linux you are still better off using git that was packaged by the vendor.
Windows
For Windows there will be a separate video and article, but I'd recommend downloading git from git-scm.
Apple Mac OSX
For Mac OSX you could also use the package from git-scm, but probably a better way is to first install Homebrew and then that install git using:
brew install git
In case you are not familiar with it, Homebrew is a package management system for Mac OSX. It is like apt or yum for Linux. It's a tool to install all kinds of open source projects. You'll probably need a lot more tools than just git, so that's why it is probably better to use Homebrew for git as well.
timestamp: 2020-05-01T05:00:03
Download and install Git on MS Windows
Find more via the Git slides.
timestamp: 2020-05-11T18:30:01
Getting started with Git on MS Windows
Find more via the Git slides.
timestamp: 2020-05-13T20:30:01
Creating a local git repository
timestamp: 2020-05-15T06:00:02
Adding first file to your git repository
timestamp: 2022-08-26T12:00:01
Getting help for git
timestamp: 2022-09-09T10:00:07
Exercise git session 1
timestamp: 2022-09-11T08:00:06
Git file status
timestamp: 2022-09-13T10:00:10
Recording changes in Git
timestamp: 2022-09-14T11:00:11
Other
Why Git is better than Subversion for Open Source Projects
For a long time while I was still using Subversion I was bombarded by people wanting me to switch to Git. It took me quite some time to understand the value in it. Let me tell you my story.
I ran a mid-sized open source project that had around 100 contributors throughout its life.
We used Subversion on the project server as our public version control system and we used Trac as our bug tracking system. Trac had a nice integration with Subversion. We could mention a Trac ticket number in our commit comment, or even in the comments of our code and Trac would display our source code and our commits in a nice way (hyper)linking those bits to the relevant tickets. We could also mention commit numbers in our bug-reports or in the comments of our bug reports and those were automatically linked to the relevant commits.
So it was all really nice.
Why Subversion and not Git?
However the main reason we used Subversion was that when I started the project Git and GitHub were very young, and I did not know how and why to use either of those. Then we kept using Subversion out of inertia.
In our setup if someone wanted to contribute some code we had two choices:
- External contributor: Let the person create a patch, send it to us (or attach it to a bug report) and then wait till we apply it. We had to go through some rather painful manual process to apply the patch. If we wanted the patch to be improved we would need to comment on the patch, the contributor would need to make changes and then we had to review the whole change again. The contributor had to include a full change in one diff. It was not easy to send several small patches and also to review them. If the same contributor wanted to work on some other change that relied on the first one being accepted, s/he had to manage the possible changes in the first patch.... A headache.
- Collaborator, or core contributor: The other possibility was to give the person commit rights on our Subversion repository. For this first the person had to create an account on our Trac and then one of the core developers had to give commit bit. This of course is a huge risk. If the contributor has different coding style, or different quality expectations, to put it nicely, then we opened the whole project up for that risk. Taking away such rights is very unpleasant. I am not sure if it is possible at all without creating a huge mess and tension in the project.
In other words in this model, we had to make people "core contributors" just to make it easy for them to contribute. We had a hard time keeping people as "external contributors" without causing them and ourselves massive pain by the process.
Git and GitHub to the rescue
After I've started to use Git and more specifically GitHub, I understood that it had a much better model.
As Ahmad M. Zawawi pointed out to me, it makes it easy to offer different rights to collaborators (core developers) and contributors of an open source project.
Only the core developers, and in many open source projects it means only one person, need to have commit rights on the main repository of the project.
Any other contributor can, without requesting any permission, make changes to the project and send you as many "patches" as she wants. Each "patch" can be a series of small commits that is much easier to develop and is usually much easier to review than one large change. In GitHub terminology, these "patches" are called "pull-requests".
The contributor can even create several changes each one depending on the other one and if you ask for some improvements in
one of the changes, Git makes is very easy for the contributors to reorganize (rebase
) the subsequent changes.
In other words, GitHub makes it very easy for the "drive-by contributors" to come in, suggest some changes and leave. Without a lot of extra administrative work. It also makes it very easy for the more persistent contributors to stick around and help with the project without you giving them any extra privileges on the project.
From the point of view of the core-developers, GitHub makes it very easy to merge a change offered by one of the contributors ("accept a pull request" in GitHub-speak), or to ask for some refinement in the recommended changes.
GitHub also offers integrated bug tracking with all the nice features we had in Trac and much more.
Back to Padre
At one point Kaare Rasmussen made a huge effort and moved Padre to GitHub, but unfortunately that was too late for the project. By that time, most of the contributors have lost interest in the project, and new people have not stepped in their place.
The code is still there, people are still using Padre. There are a number of stand-alone module such as Parse::ErrorString::Perl and Debug::Client that came out of the project.
Unfortunately, however, the momentum is gone.
Conclusion
Git and GitHub are way better for Open Source development than Subversion was.
timestamp: 2016-05-13T15:30:01